Mastering Python: A Comprehensive Guide
InfoThis is a summary of the following YouTube video:
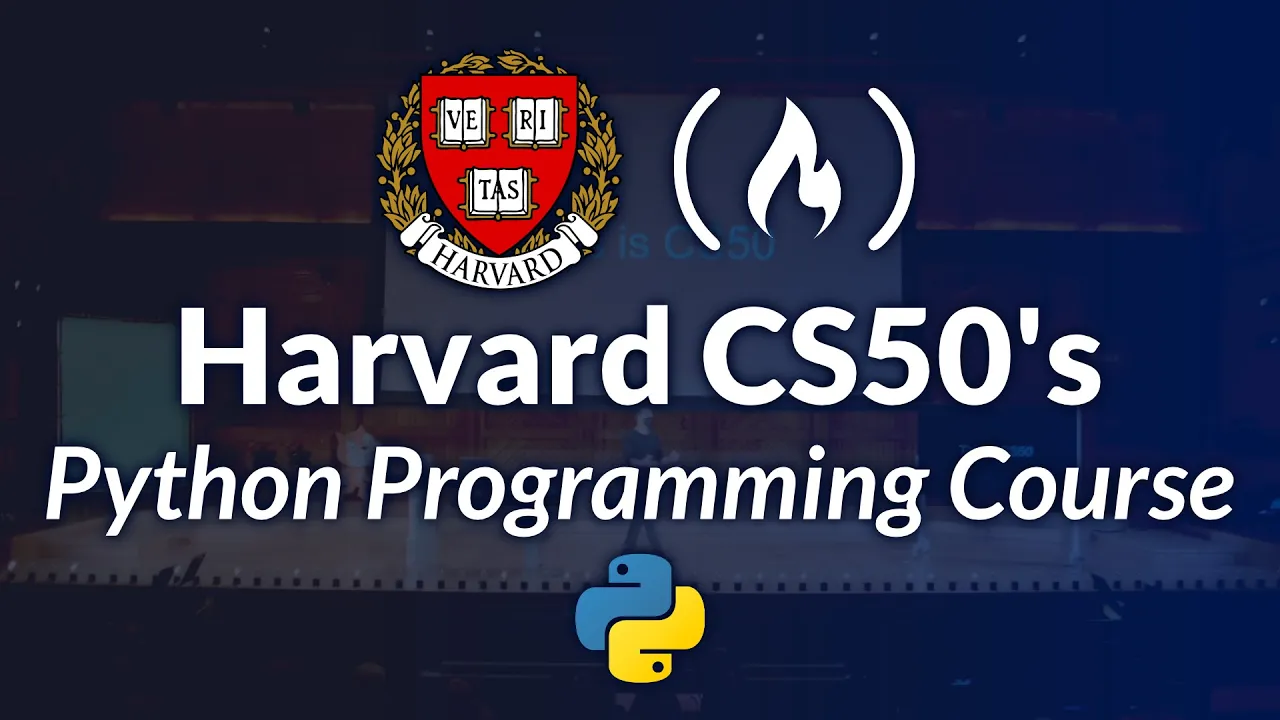
Harvard CS50’s Introduction to Programming with Python – Full University Course
freeCodeCamp.org
May 1, 2023
·
Education
Comprehensive Python programming course
- The course is an introduction to programming using Python, offered by Harvard University.
- It is taught by Dr. David Malan and is designed for students with or without prior programming experience.
- The course covers fundamental programming concepts such as functions, variables, conditionals, loops, exceptions, libraries, unit tests, file I/O, regular expressions, and object-oriented programming.
- Students will learn how to read, write, test, and debug code.
- The course includes hands-on opportunities and exercises inspired by real-world programming problems.
- No specific software is required; a web browser or any text editor like Google Docs or Microsoft Word can be used.
- The course can be taken before, during, or after the original CS50 course, which covers broader computer science topics.
- The course emphasizes practical skills and provides tools and vocabulary for solving real-world problems in various fields.
Debugging and improving Python code
- When writing a program for the first time, it may not work initially, but with time, practice, and experience, it will improve.
- Even minor details like a missing parenthesis can cause a program to fail, as computers require precise instructions.
- Using a text editor like VS Code is recommended for writing code, as it provides features like syntax highlighting and debugging tools.
- Running Python programs via the terminal window is useful for learning, but for broader use, a graphical user interface (GUI) can be developed.
- To make a program interactive, you can use the input function to prompt the user for input and store it in a variable.
- Variables in programming act as containers for values, allowing you to store and reuse user input.
- The assignment operator (=) in Python assigns the value from the right side to the variable on the left side.
- Printing the value of a variable requires passing the variable to the print function without quotes.
- Improving a program involves making it more user-friendly and interactive, such as personalizing greetings based on user input.
Importance of comments and pseudocode
- As programs grow longer, they can become dozens or even hundreds of lines long. To manage this complexity, Python and many other programming languages support comments, which are notes to yourself in the code. Comments are ignored by the computer and are meant for the programmer or anyone else reading the code.
- In Python, comments are typically added using the hash symbol (#). Comments can be used to explain what a section of code does, making it easier to understand when revisiting the code later. This is especially useful for debugging or when sharing code with others.
- Comments can also serve as a to-do list for the programmer. This practice is known as pseudocode, which is a way of outlining your program in plain language before writing the actual code. Pseudocode helps break down a larger problem into smaller, manageable tasks.
- The 'input' function in Python is used to get user input, which is always returned as a string. This means it expects text, regardless of the language used.
- For multi-line comments, Python supports using triple quotes (''' or """). This allows for comments that span multiple lines, which can be useful for more detailed explanations.
- The 'print' function in Python can take multiple arguments, separated by commas. This automatically inserts a space between the arguments when printed. Alternatively, the plus operator (+) can be used to concatenate strings, but this does not add a space between them.
- The discussion also touched on the concept of functions and how they can be reused to solve recurring problems in code. If a problem is encountered multiple times, it is efficient to create a custom function to handle it.
- The term 'string' in Python refers to a sequence of text characters and is often abbreviated as 'str'. This is a specific type of data in programming, and understanding different data types is crucial for effective coding.
Understanding Python's print function
- The user initially used two print statements to print 'hello' and 'name' separately, which resulted in an undesirable visual effect with text on separate lines.
- The print function in Python automatically moves the cursor to the next line after printing, which caused 'hello' and 'David' to appear on different lines.
- To understand and control this behavior, the user referred to Python's official documentation, which explains the parameters and arguments of the print function.
- The print function can take multiple arguments, and by default, it separates them with a space and ends with a newline character ('\n').
- The 'sep' parameter in the print function specifies the separator between multiple arguments, and the 'end' parameter specifies what to print at the end of the output.
- By setting 'end' to an empty string (''), the user can prevent the print function from moving to the next line, keeping 'hello' and 'David' on the same line.
- The user demonstrated this by modifying the print statement to include 'end=""', which successfully kept the output on the same line.
- The user also experimented with the 'sep' parameter to change the default space separator to a different character, such as '???', to illustrate its effect.
- The user explained the difference between parameters (what a function can take) and arguments (what is actually passed to the function).
- The user introduced the concept of escape characters, such as '\n' for newline and '\"' for including quotes within a string.
- The user demonstrated how to use single quotes and escape characters to include double quotes within a string.
- The user introduced format strings (f-strings) in Python, which allow embedding expressions inside string literals using curly braces and an 'f' prefix.
- The user showed how to use f-strings to include variable values directly within a string, providing a more elegant solution for formatting output.
Python string manipulation methods
- The text discusses the importance of handling user input in programming, particularly focusing on the common issue of users accidentally adding spaces or not capitalizing words properly.
- It introduces the concept of built-in string methods in Python, such as 'strip', which removes whitespace from the beginning and end of a string, and 'capitalize', which capitalizes the first letter of a string.
- The text explains how to use these methods by chaining them together to clean and format user input more efficiently. For example, using 'name.strip().title()' to remove extra spaces and capitalize the first letter of each word in a user's input.
- It highlights the importance of reassigning the cleaned and formatted string back to the original variable to ensure the changes are saved.
- The text also touches on the readability and maintainability of code, discussing the trade-offs between writing concise code and breaking it into multiple lines for clarity.
- A question-and-answer session is included, where participants discuss the limitations of the 'strip' method and the potential for chaining multiple methods together, as well as the pros and cons of different coding styles.
Python programming basics and interactive mode
- The process of improving programming skills involves developing a personal style or adhering to company standards. It's important to have a reasoned argument for your coding choices, even if others disagree.
- Strings in Python have various methods, including 'split', which can divide a string into substrings based on a specified delimiter. This is useful for tasks like separating a first name from a last name.
- Python supports integers (int), which are whole numbers without decimal points. Basic arithmetic operations like addition, subtraction, multiplication, and division are supported, along with the modulo operator for finding remainders.
- Python offers an interactive mode where you can execute code line-by-line without saving it to a file. This mode is useful for quick calculations and testing small code snippets.
- Creating a simple calculator in Python involves using variables, getting user input, and performing arithmetic operations. User input is initially treated as a string and must be converted to an integer for mathematical operations.
- The 'int' function in Python converts strings that look like numbers into actual integers, allowing for proper arithmetic operations.
- Nested functions in Python allow for more concise code by using the return value of one function as the input to another. This can simplify operations like converting user input to integers and then adding them.
Python function nesting and readability
- The text explains the concept of evaluating innermost parentheses first in both mathematics and programming. Python evaluates expressions inside parentheses first, then passes the return value to the outer function.
- An example is given where the input function is called first, and its result is passed to the int function. This process is repeated for multiple lines of code to convert strings to integers before performing arithmetic operations.
- The text discusses the benefits of removing unnecessary variables to simplify code. It shows how to eliminate a variable by directly printing the sum of two integers, making the code cleaner and more readable.
- A debate is presented on whether the new version of the code, which eliminates a variable, is better than the old version. Arguments for the new version include improved readability and reduced clutter, while arguments for the old version include easier debugging.
- The importance of handling errors is highlighted. The text mentions that future lessons will cover how to handle cases where the user inputs non-integer values, such as words, which cannot be converted to integers.
- The text emphasizes the importance of code readability and simplicity. It advises against making code too complex or clever, as this increases the likelihood of errors and makes the code harder to understand and maintain.
- An example is given where the code is simplified to a single line, but this is criticized for being less readable. The text argues that breaking code into multiple lines can make it easier to understand and debug.
- The concept of floating-point values is introduced. The text explains how to modify the code to handle decimal numbers by converting input values to floats instead of integers.
- The round function is introduced to round floating-point results to the nearest integer. The text explains how to use the round function and its optional parameter to specify the number of decimal places.
- The text discusses formatting numbers with commas for better readability. It introduces the F string in Python and shows how to use it to format numbers with commas, making large numbers easier to read.
Python floats have precision limits
- The text discusses the automatic formatting of numbers in Python, which can vary based on locale settings. It highlights the use of periods and commas in different countries for number formatting.
- A question is raised about the precision of floating-point numbers in Python. The response explains that floats cannot represent numbers infinitely precisely due to finite memory in computers, leading to eventual rounding.
- An example of simple division using floats is provided, showing how Python handles division and the resulting precision. It is noted that while integers in Python can be arbitrarily large, floats have a precision limit.
- The text demonstrates how to round floating-point numbers to a specific number of decimal places using the round function and format strings. It explains the syntax for specifying the number of digits in an f-string.
- The concept of defining custom functions in Python is introduced. The text walks through creating a simple function called 'hello' that prints a greeting. It explains the use of the 'def' keyword and the importance of indentation.
- The text further elaborates on customizing functions with parameters. An example is given where the 'hello' function is modified to take a name as an argument, allowing for personalized greetings.
Python function definition and usage
- The text explains how to define a function in Python with default parameter values. It uses the example of a 'hello' function that greets the user, with a default parameter value of 'world'.
- It demonstrates how to call the 'hello' function with and without arguments, showing the output for both cases. The importance of defining functions before calling them is emphasized, as Python's interpreter requires functions to be defined before they are used.
- The text discusses the concept of 'scope', explaining that variables defined within a function are not accessible outside of that function. This is illustrated with an example where a variable 'name' is defined in the 'main' function but is not accessible in the 'hello' function.
- To address the issue of function definition order, the text introduces the convention of defining a 'main' function that contains the main logic of the program. This 'main' function is then called at the end of the file, ensuring that all necessary functions are defined before they are used.
- The text also covers the use of the 'return' keyword to return values from functions. An example is provided where a 'square' function is defined to return the square of a given number. Different ways to implement the squaring operation are discussed, including using the '**' operator and the 'pow' function.
- The importance of avoiding code repetition by defining reusable functions is highlighted. The text explains that defining functions helps to organize code logically and makes it easier to read and maintain.
- Finally, the text summarizes the key concepts covered: defining functions, using default parameter values, understanding variable scope, organizing code with a 'main' function, and using the 'return' keyword to return values from functions.
Python conditional logic and comparison
- Python provides built-in syntax for making logical decisions in code, using symbols like >, >=, <, <=, ==, and != to compare values.
- The 'if' keyword in Python allows you to execute code based on whether a condition is true. For example, 'if x < y' will execute a block of code if x is less than y.
- Indentation is crucial in Python; it indicates which code block should be executed if the condition is true. A colon (:) follows the 'if' statement to signify the start of an indented block.
- Boolean expressions, named after mathematician George Boole, are questions that have true or false answers. These are used in 'if' statements to control the flow of the program.
- Flowcharts can visually represent the logic of a program, showing how different conditions lead to different outcomes. This helps in understanding the control flow of the program.
- Using 'elif' (else if) in Python allows for more efficient code by making conditions mutually exclusive. This means once a true condition is found, the subsequent conditions are not checked.
- The example program 'compare.py' demonstrates how to use 'if', 'elif', and 'else' to compare two user-input integers and print whether one is less than, greater than, or equal to the other.
- The program's logic can be visualized using a flowchart, which shows the decision-making process and the control flow based on different conditions.
- Efficient code design is important for long-term maintenance and performance, even if the immediate performance gains are not noticeable.
Simplifying conditional logic
- The text begins with a comparison of two values, X and Y, using a flowchart to determine if X is less than, greater than, or equal to Y. The initial approach involves asking multiple questions to reach a conclusion.
- The first improvement discussed is the use of 'else' to simplify the logic. Instead of asking if X is equal to Y after checking if X is less than or greater than Y, the 'else' statement assumes X must be equal to Y if the other conditions are false.
- The text explains that using 'else' reduces the number of questions asked, making the code more efficient and easier to read. This simplification is illustrated with a revised flowchart that has fewer nodes and arrows.
- A further refinement is introduced by using the 'or' operator to combine conditions. The code checks if X is less than or greater than Y in one statement, printing that X is not equal to Y if either condition is true, and using 'else' to print that X is equal to Y.
- The final optimization involves directly checking if X is not equal to Y, which simplifies the code even more. If X is not equal to Y, it prints that X is not equal to Y; otherwise, it prints that X is equal to Y.
- The text emphasizes the importance of writing concise and readable code, highlighting that fewer lines of code reduce the likelihood of errors and make the code easier for others to understand.
- The discussion includes a brief explanation of Python's indentation and colon requirements, noting that proper indentation is crucial for the code to work correctly in Python.
- The text concludes with an introduction to the 'and' operator, suggesting its use for combining multiple conditions in a single statement, and hints at further exploration of this concept in a new program.
Python grading logic optimization
- The text begins by explaining how to get a user's score and store it in a variable called 'score' using the 'int' function to convert the user's input into an integer.
- It describes the common grading system in the U.S., where scores between 90-100 are an A, 80-89 are a B, 70-79 are a C, 60-69 are a D, and below 60 is an F. The text then shows how to use conditionals to assign grades based on these ranges.
- The initial approach uses multiple 'if' and 'elif' statements to check if the score falls within specific ranges and prints the corresponding grade. The text highlights the importance of ensuring that the boundaries between grades are correct.
- The text then suggests an optimization by flipping the comparison operators and combining the range checks into a single line using Python's ability to chain comparisons. This makes the code more succinct and easier to read.
- Further optimization is proposed by reducing the number of questions asked. Instead of checking both the lower and upper bounds for each grade range, the code only checks if the score is greater than or equal to the lower bound, assuming the input is between 0 and 100. This makes the code more efficient and maintainable.
- The text also discusses the potential pitfalls of not using 'elif' and how it can lead to unintended results, as each condition would be checked independently, causing multiple grades to be assigned incorrectly.
- The text concludes by introducing the modulo operator (%) to determine if a number is even or odd. It explains how the modulo operator can be used to check if a number is divisible by 2 without a remainder, which indicates that the number is even. If there is a remainder, the number is odd.
Python function creation and optimization
- The speaker demonstrates how to determine if a number is even or odd using Python code. They start by manually checking numbers and then propose creating a function to automate this process.
- A main function is defined to get user input and call a hypothetical 'is_even' function. The speaker explains the importance of assuming the existence of functions during problem-solving, even if they haven't been created yet.
- The 'is_even' function is defined to take a number as an argument and return a Boolean value. The speaker explains the concept of Boolean values in Python, which can only be true or false.
- The function checks if the number is divisible by 2 without a remainder to determine if it is even, returning true if it is and false otherwise. This function is then used in the main function to print whether the number is even or odd.
- The speaker answers questions about Python's handling of arguments and the use of functions. They clarify that Python does not support passing arguments by address like Java or C++ and explain the use of built-in string functions.
- The speaker introduces the concept of writing 'pythonic' code, which is more concise and readable. They demonstrate how to condense the 'is_even' function from multiple lines to a single line using a more elegant syntax.
- Further optimization is shown by directly returning the Boolean expression without using an if-else statement. This makes the code more succinct while maintaining readability.
- The speaker transitions to a new example using a 'match' statement, similar to a 'switch' statement in other languages, to determine a Harry Potter character's house based on their name. They demonstrate the traditional if-elif-else construct first.
- The program prompts the user for a name and prints the corresponding house or a default message if the name is not recognized. The speaker runs the program with different inputs to show its functionality.
- The speaker hints at further improvements and optimizations that can be made to the code, emphasizing the importance of writing clear and efficient code.
Python match statement simplifies conditional logic
- The speaker discusses optimizing code that checks if characters Harry, Hermione, or Ron are in Gryffindor by consolidating multiple 'if' statements into one using the 'or' keyword.
- The speaker demonstrates running the program with different inputs (Hermione, Ron) to verify the correctness of the consolidated 'if' statement.
- The speaker introduces the 'match' statement as a more compact and less verbose alternative to multiple 'if-elif-else' statements, especially useful when handling many cases.
- The 'match' statement syntax is explained, showing how to handle specific cases (Harry, Hermione, Ron, Draco) and a catch-all case using an underscore for unspecified names.
- The speaker runs the program again to verify the correctness of the 'match' statement implementation with various inputs (Harry, Hermione, Ron, Draco, Padma).
- The speaker further optimizes the 'match' statement by combining multiple cases (Harry, Hermione, Ron) into a single line using vertical bars, making the code more concise.
- The speaker emphasizes that there are multiple ways to solve programming problems and that the 'match' statement is a useful tool for simplifying conditional logic.
- The speaker transitions to discussing loops, specifically the 'while' loop, to avoid repetitive code and make the program more efficient.
- An example is given where a 'while' loop is used to print 'meow' three times, demonstrating how to avoid code duplication and improve maintainability.
- The speaker explains the importance of avoiding repetitive code and introduces the 'while' loop as a solution to perform repetitive tasks efficiently.
Python loops and control flow
- The text begins by initializing a variable I to 3 and explaining the concept of a while loop. The loop continues to execute as long as the condition (I does not equal 0) is true. This results in an infinite loop if the value of I is not changed within the loop.
- To avoid an infinite loop, the value of I is decremented by 1 in each iteration. This ensures that the loop eventually terminates when I reaches 0. The text also provides a tip to use Ctrl+C to interrupt an infinite loop in the terminal.
- A flowchart is introduced to visually represent the while loop's logic. The flowchart shows the initialization of I, the condition check, the action (printing 'meow'), and the update of I. This helps in understanding the loop's structure and flow.
- An alternative approach is discussed where the loop counts up from 1 to 3 instead of counting down from 3 to 0. The condition is changed to check if I is less than or equal to 3, and I is incremented by 1 in each iteration. This approach is more intuitive for some people.
- The text explains the convention of starting loops from 0 in programming. The loop is modified to start from 0 and go up to but not including 3. The condition is changed to I < 3, and the increment operation is simplified using the += operator.
- The text introduces the concept of a for loop as an alternative to the while loop. A for loop iterates over a list of values, making the code shorter and more readable. The example provided shows a for loop iterating over the list [0, 1, 2] and printing 'meow' in each iteration.
- The text briefly mentions the use of lists in Python, which allows for containing multiple values in a single variable. This is demonstrated in the context of the for loop, where the list [0, 1, 2] is used to control the loop's iterations.
Efficient Python coding techniques
- The text discusses the use of lists in Python, specifically how to create and use them with square brackets. Lists are defined using square brackets, which visually and syntactically indicate a list to both the programmer and Python.
- The example provided demonstrates a simple program that prints 'meow' three times using a list. The program is functional but not optimally designed, as it manually specifies the list of values (0, 1, 2). This approach is impractical for larger lists, such as printing 'meow' a million times.
- To improve the design, the text suggests using Python's built-in 'range' function, which generates a sequence of numbers. This function simplifies the code and makes it scalable, allowing the program to handle larger sequences without manually specifying each value.
- The text introduces a Pythonic convention of using an underscore (_) as a variable name when the variable's value is not needed. This practice improves code readability and signals to others that the variable is only used for iteration purposes.
- An advanced technique is shown where the 'print' function is used with string multiplication to print 'meow' multiple times. By multiplying the string 'meow' by a number, the program can print it that many times. However, this method initially results in all 'meows' appearing on the same line.
- To address the formatting issue, the text suggests using the newline character '\n' within the string to ensure each 'meow' appears on a new line. Additionally, the 'end' parameter of the 'print' function is adjusted to prevent an extra blank line at the end of the output.
- The text emphasizes the importance of writing readable and maintainable code. While the advanced techniques are efficient, they should not compromise the readability of the code. The simpler for-loop approach is also valid and may be preferable in some cases.
- The text concludes with an example of using a while loop to repeatedly prompt the user for input until a valid positive number is provided. This demonstrates a common pattern in Python for handling user input and ensuring it meets specific criteria.
Python function creation and list iteration
- The text begins with an example of creating a custom function in Python. The author defines a 'main' function and calls a non-existent 'meow' function, which is then defined to print 'meow' a specified number of times.
- The 'meow' function takes an input parameter 'n' and uses a for loop to print 'meow' 'n' times. The 'main' function is called to execute the code, and the hardcoded value '3' is used initially.
- To improve the code, the author introduces user input by defining a 'get_number' function. This function repeatedly prompts the user for a positive number and returns it once a valid input is received.
- The 'get_number' function uses a while loop to ensure the input is a positive integer. It demonstrates the use of the 'return' keyword to exit the loop and return the value to the caller.
- The author then replaces the hardcoded '3' in the 'main' function with the value obtained from 'get_number', allowing the user to specify how many times 'meow' is printed.
- The text transitions to discussing lists in Python. The author creates a list of students from Hogwarts and explains how to print each student's name individually using list indexing.
- The author demonstrates how to use a for loop to iterate over the list of students and print each name. This method is more efficient and adaptable to changes in the list's length.
- The text explains the concept of zero-based indexing in Python, where the first item in a list is at index 0. This is illustrated by printing each student's name using their index.
- The author shows how to use a for loop with the 'range' function and the 'len' function to iterate over the list by index. This method is useful when the program requires numerical iteration.
- The text concludes with an example of printing both the index and the student's name, demonstrating how to combine multiple elements in a print statement.
Python dictionaries associate keys with values
- The text begins with a list of students ranked by their performance: Hermione is first, Harry is second, and Ron is third. This list is used to demonstrate how to access values in Python lists.
- A question is raised about the 'for i in range' loop, prompting a detailed explanation. The loop creates a variable 'i' and assigns it values from a list, executing indented code for each value. The 'range' function is introduced to handle larger iterations efficiently.
- The example transitions to using the 'length' function to determine the number of students dynamically, avoiding hardcoding values. This demonstrates composing multiple ideas to create flexible code.
- The text introduces Python dictionaries, a data structure that associates keys with values, similar to words and definitions in a human dictionary. This is more powerful than lists, which only store multiple values.
- An example is given to track students' houses at Hogwarts using lists, but it is noted that this approach becomes cumbersome with more data. The solution is to use dictionaries to associate students with their houses.
- The syntax for creating and using dictionaries is explained. Curly braces are used to define dictionaries, and key-value pairs are added. The example shows associating students with their houses using a dictionary.
- The text demonstrates how to print values from a dictionary by using keys. It shows how to iterate over dictionary keys using a 'for' loop and access corresponding values, making the code more dynamic and readable.
- The example is extended to print both keys and values in a formatted manner, improving readability. The 'print' function's separator feature is used to format the output neatly.
- A question is addressed about how the 'for student in students' loop works with dictionaries. It is explained that the loop iterates over dictionary keys, setting the loop variable to each key in turn.
- The text concludes with a more complex example, suggesting that additional information about students can be managed using dictionaries, highlighting the flexibility and power of this data structure.
Using Python dictionaries for complex data
- The text discusses implementing a program to manage data about students at Hogwarts, including their names, houses, and Patronuses.
- It introduces the concept of using a list of dictionaries to store multiple pieces of information about each student, such as their name, house, and Patronus.
- The example provided includes creating a list of dictionaries for four students: Hermione, Harry, Ron, and Draco, each with their respective attributes.
- The text explains how to define and initialize dictionaries in Python, using curly braces and key-value pairs.
- It highlights the use of the special keyword 'None' in Python to represent the absence of a value, as demonstrated with Draco's Patronus.
- The text demonstrates how to iterate over a list of dictionaries using a for loop to access and print specific information about each student.
- It also covers how to format the output for better readability by adding separators between values.
- The text briefly touches on the efficiency of Python dictionaries and their ability to quickly retrieve data, even from large datasets.
- Finally, it transitions to a different example, using a simple game-inspired scenario to illustrate the use of loops and functions in Python.
Functions simplify code reuse
- The speaker discusses the concept of functions in programming, using the example of a function called 'print column' to print a part of a game world. Functions allow for abstraction and code reuse, making it easier to change the implementation without affecting other parts of the code.
- The speaker demonstrates how to re-implement the 'print column' function using different methods, such as string multiplication. This shows the flexibility of functions, as the main code does not need to know about the changes in the function's implementation.
- The speaker transitions to printing horizontal rows instead of vertical columns, introducing a new function called 'print row'. This function takes a width parameter and uses string multiplication to print a row of question marks. This demonstrates the concept of abstraction in problem-solving.
- The speaker explains the importance of abstraction in programming, using the example of printing a 3x3 grid in a game. They introduce a function called 'print square' that uses nested loops to print the grid, emphasizing the need to think two-dimensionally when dealing with such problems.
- The speaker provides a detailed explanation of how nested loops work, comparing the process to an old-school typewriter or printer that prints from top to bottom and left to right. This helps in understanding the logic behind generating a 2D output.
- The speaker simplifies the 'print square' function by using string multiplication instead of nested loops, making the code more compact and easier to understand. They also introduce the concept of breaking down a larger program into smaller, manageable functions.
- The speaker concludes the discussion on loops in Python, highlighting the importance of combining loops with conditionals, functions, and variables to solve complex problems. They hint at future topics, such as handling exceptions in Python.
Handling errors in Python
- The speaker discusses common mistakes in Python programming, such as forgetting to close a quote, which leads to syntax errors. Syntax errors must be fixed by the programmer as they indicate issues with the code's structure.
- The speaker demonstrates running a Python script and encountering a syntax error due to an unterminated string literal. The error message helps identify the problem, which is fixed by closing the quote.
- Syntax errors are contrasted with runtime errors, which occur while the code is running. The speaker emphasizes the importance of writing defensive code to handle unexpected inputs from users.
- A new example is introduced where the speaker writes a Python script to prompt the user for an integer and print it. The importance of converting user input to an integer using the int function is highlighted.
- The speaker tests the script with various inputs, including positive numbers, zero, negative numbers, and a string. The string input causes a value error, demonstrating the need for error handling.
- The concept of error handling in Python is introduced using the try and except keywords. The speaker modifies the script to catch value errors and print an appropriate error message when the input is not an integer.
- The importance of handling specific errors rather than catching all errors is discussed. Catching all errors can hide bugs and is considered bad practice. The speaker advises identifying and handling specific errors explicitly.
Handling exceptions in Python
- The text discusses improving code by handling exceptions more elegantly, rather than relying on default Python error messages.
- It emphasizes the importance of isolating the minimal amount of code that can raise an exception within a try block to follow best practices.
- The author explains a common mistake where a NameError occurs because a variable is not defined due to an exception interrupting the assignment process.
- The text introduces the use of the else clause in try-except blocks to handle code execution when no exceptions occur, similar to its use in conditionals.
- An example is provided where a loop is used to repeatedly prompt the user for input until a valid integer is provided, demonstrating a more user-friendly approach.
- The author walks through the control flow of the program, explaining how the loop and exception handling work together to ensure correct input is received.
Using break and return in loops
- The else clause in Python's try-except-else structure is associated with the try block, not the except block. This means that the else block will execute only if no exceptions are raised in the try block.
- When handling user input in a loop, using break allows you to exit the loop once the desired condition is met. For example, if you are prompting the user for an integer, you can use break to exit the loop once a valid integer is entered.
- If you place a print statement inside the loop without a break, the loop will continue indefinitely, printing the statement each time. This can be useful in some scenarios but is generally not desired if you want the loop to terminate after a certain condition is met.
- You can use the break statement inside various control structures like if, elif, else, and try-except-else. This flexibility allows you to handle different scenarios and exit loops based on specific conditions.
- In the context of exception handling, you can place the break statement inside the else block or directly after the try block if no exceptions are raised. This ensures that the loop exits only when the desired condition is met without any errors.
- Creating a function like get_int to handle repetitive tasks such as getting an integer input from the user can make your code more modular and reusable. This function can include exception handling to ensure that the input is a valid integer.
- Using return in a function not only exits the loop but also returns a value from the function. This is more efficient than using break followed by return, as it combines both actions into a single statement.
- You can further simplify your code by directly returning the result of an expression, such as return int(input('What’s X?')), instead of assigning it to a variable first. This reduces the number of lines and potential errors.
- The pass statement in Python allows you to catch an exception without handling it. This can be useful if you want to ignore certain errors and continue executing the rest of the code. For example, you can use pass to silently ignore invalid inputs and keep prompting the user for a valid input.
- The design of your code should balance readability and efficiency. While shorter code can be easier to read and less prone to errors, it should also be clear and understandable. Making conscious decisions about code structure and justifying them is important for good programming practice.
Python indentation is crucial
- Indentation in Python is deliberate and logical, unlike some other languages that use curly braces or other symbols to denote code blocks. Indentation in Python signifies that the indented lines are associated with the preceding line and should only be executed if the preceding line's condition is met.
- For example, in a function definition, all lines indented under the function header are part of that function. Similarly, lines indented under a 'while' loop are part of that loop and will be executed repeatedly as long as the loop's condition is true.
- The 'try' and 'except' blocks in Python also use indentation to indicate which lines of code should be tried and which should be executed if an exception occurs. This makes the code more readable and helps in understanding the logic of the program.
- Handling errors in Python can be done using 'try' and 'except' blocks. If an error is handled within these blocks, the caller will not know about the error. Functions like 'isnumeric' can be used to check if a string is a number before converting it to an integer, but the Pythonic way is to try the conversion and handle any exceptions that occur.
- To make functions more reusable, parameters can be added to functions so that they do not rely on hardcoded values. For example, a function can take a 'prompt' parameter to display different messages to the user, making the function more flexible and reusable.
- Python supports the use of libraries or modules, which are files of code that can be reused across different programs. Modules like 'random' provide additional functions that are not available by default and need to be imported using the 'import' keyword.
- The 'random' module, for example, contains functions like 'choice' that can be used to select a random element from a list. This promotes code reusability and helps avoid duplicating code across different projects.
Python's random module for probability
- The text explains how to use Python's random module to simulate a coin toss using the random.choice function. It describes how random.choice takes a list and returns one of the values with equal probability. The example demonstrates storing the result in a variable and printing it to observe the outcomes.
- The text introduces the from keyword in Python, which allows importing specific functions from a module. This is contrasted with the import keyword, which imports the entire module. The example shows how using from random import choice simplifies the code by allowing direct use of the choice function without prefixing it with the module name.
- The text discusses the advantages of using from to import specific functions, such as avoiding name conflicts and making the code cleaner. It also mentions that in older programming languages, importing libraries could lead to conflicts if the same function or variable names were used.
- The text introduces another function from the random module, randint, which generates a random integer between two specified values, inclusive. The example demonstrates generating random numbers between 1 and 10 and printing them to observe the distribution.
- The text explains the shuffle function from the random module, which randomizes the order of elements in a list. The example shows shuffling a list of cards and printing each card in the new order using a for loop. It highlights that shuffle modifies the list in place without returning a new list.
- The text addresses a question about adjusting probabilities, explaining that while the basic functions in the random module provide equal probabilities, more sophisticated functions or custom implementations can be used to control probabilities. It cautions against manipulating probabilities in contexts like gambling games.
- The text briefly mentions the statistics module in Python, which includes functions for statistical analysis, such as calculating means, medians, and modes. It provides an example of using the statistics.mean function to calculate the average of a list of numbers.
Python command line arguments
- The speaker discusses calculating the average of two test scores using Python's statistics module. They use the mean function to compute the average and print the result, which is 95 percent.
- The speaker introduces the concept of command line arguments, which allow users to provide input to a program at the command line rather than through prompts within the program. This feature is common in many programming languages, not just Python.
- To demonstrate command line arguments, the speaker creates a Python file named name.py and uses the sys module to access command line arguments. The sys module contains a variable called sys.argv, which is a list of all the words typed at the command line.
- The speaker explains that sys.argv[0] contains the name of the program being executed, while sys.argv[1] and beyond contain any additional arguments provided by the user. They demonstrate this by writing a program that prints a name tag using the command line argument as the name.
- The speaker runs the program with the command 'python name.py David' and shows that it prints 'Hello, my name is David.' They explain that if no name is provided, the program will raise an IndexError because sys.argv[1] does not exist.
- To handle this error, the speaker suggests using a try-except block to catch the IndexError and print a user-friendly message. They also propose using conditionals to check the length of sys.argv and provide appropriate error messages if there are too few or too many arguments.
- The speaker demonstrates the improved program, which now checks the number of command line arguments and prints 'Too few arguments' or 'Too many arguments' as needed. If the correct number of arguments is provided, it prints the name tag as intended.
- The speaker emphasizes the importance of reading documentation and anticipating potential errors to write more robust code. They encourage handling exceptions and validating input to improve the user experience and prevent crashes.
Handling command-line arguments
- The speaker demonstrates running a Python script with different command-line arguments to handle errors like 'too few' or 'too many' arguments. They show how to print a name tag based on the input provided.
- They explain the importance of using conditionals to check for errors before proceeding with the main logic of the program. This helps in providing more refined feedback to the user rather than generic error messages.
- A question is raised about handling full names and separating first and last names. The speaker explains how to use quotes to handle full names as a single argument and suggests that the program can be adapted to support multiple names.
- The speaker discusses the importance of separating error handling from the main logic of the program for better code design. They demonstrate how to use the 'sys' module to exit the program early if there are errors, ensuring that the main logic only runs when the input is correct.
- A question is asked about using multiple 'else' statements, to which the speaker clarifies that only one 'else' statement is allowed, but multiple 'elif' statements can be used for different conditions.
- The speaker refines the code to handle multiple names by iterating over the command-line arguments using a 'for' loop. They explain how to use slicing to exclude the program's name from the list of arguments.
- A question is raised about accessing specific elements in the argument list, and the speaker explains how to use slicing to get subsets of the list. They demonstrate how to print name tags for multiple names provided as arguments.
- The speaker concludes by showing how to use slicing syntax to handle different parts of the argument list, ensuring that the program can handle various input scenarios effectively.
Python package management and usage
- Using negative indices in Python allows counting from the end of a list. This can be useful for various operations, such as slicing lists from the end.
- Python's format code in f-strings is context-specific. The colon, period, number, and letter 'F' are used for formatting numbers, which is different from using a colon for slicing.
- Python's popularity is partly due to its extensive third-party libraries, also known as packages. These packages can be installed on local machines or cloud servers to extend Python's functionality.
- The Python Package Index (PyPI) is a repository for Python packages. It can be accessed via the web or command line to download and install packages.
- The 'pip' package manager is used to install Python packages. It simplifies the process of adding new libraries to your Python environment.
- An example of a fun Python package is 'cowsay', which allows a cow to say something on the screen. This demonstrates how to install and use third-party packages.
- Command line arguments in Python can be used to customize commands and automate processes, making programming more efficient.
- APIs (Application Programming Interfaces) allow programs to interact with third-party services. Python's 'requests' library is commonly used to make web requests and retrieve data from APIs.
- Installing the 'requests' library via 'pip' allows Python programs to make HTTP requests, enabling interaction with web services like Apple's iTunes API.
Python API integration
- To specify a search for songs in a database, use 'entity=song' to ensure the search returns songs and not albums or artists.
- To limit the search to one song, use 'limit=1'. To search for a specific artist, use 'term=artist_name'. For example, 'term=Weezer' searches for songs by Weezer.
- Accessing the URL with these parameters downloads a text file containing JSON data, a standard text format for data exchange.
- JSON (JavaScript Object Notation) is language-agnostic and can be read or written using various programming languages, including Python.
- An API (Application Programming Interface) allows access to data on a server, which can be integrated into a program.
- To automate this process in Python, use the 'requests' library to make HTTP requests and the 'sys' library to handle command-line arguments.
- Error checking ensures the user provides the necessary command-line arguments (file name and band name).
- The 'requests.get' function fetches the data from the specified URL, and the response is stored in a variable.
- The response is converted to a Python dictionary using 'response.json()', which formats the JSON data into a readable structure.
- To make the JSON data more readable, use the 'json' library's 'json.dumps()' function with an 'indent' parameter for better formatting.
- Iterate over the JSON data to extract specific information, such as track names, using loops and dictionary keys.
- Adjust the 'limit' parameter to fetch more results, such as 50 songs, and print the track names in a readable format.
- Use 'sys.exit()' to terminate the program if the required command-line arguments are not provided.
Creating and using Python modules
- The keys in a JSON response from itunes.apple.com are predefined by their engineers. You can store these values in Python variables and rename them as needed.
- The Cow Say package can output various ASCII graphics, including cows and other animals. It is mainly used to demonstrate the types of packages you can install in Python.
- You can create your own Python libraries by bundling reusable code into modules or packages. This can be kept local or shared on platforms like PyPI.
- To create a Python module, define functions in a separate file and import them as needed. For example, a 'sayings' module can include 'hello' and 'goodbye' functions.
- When importing a module, use the '__name__ == "__main__"' conditional to prevent the main function from running unintentionally.
- Unit tests are essential for verifying that your code works correctly. Write tests to check various inputs and edge cases to ensure reliability.
- In the example of a calculator program, testing should include not just typical inputs but also edge cases like zero and negative numbers.
Testing Python code with assertions
- The text discusses the importance of conditionally calling the main function to avoid automatic execution when importing functions from other files.
- It explains the process of creating a separate test file, named conventionally as test_calculator.py, to test the functionality of a specific function, in this case, the square function.
- The text describes how to import the square function from the calculator file and the convention of naming test functions with a 'test_' prefix.
- Manual testing of functions is highlighted as tedious and error-prone, emphasizing the need for automated testing to ensure consistent and repeatable results.
- The use of the assert keyword in Python is introduced as a way to simplify testing by asserting that certain conditions are true, and if not, an error is raised.
- The text explains how to handle assertion errors using try and except blocks to provide more user-friendly error messages.
- It discusses the importance of testing various cases, including positive numbers, negative numbers, and zero, to ensure comprehensive coverage of potential edge cases.
- The text concludes by highlighting the balance between writing concise test code and ensuring thorough testing, suggesting that fewer lines of code can reduce the likelihood of errors.
Automate code testing with Pytest
- The text discusses the process of testing a Python function using assertions and the Pytest library.
- Initially, the author manually writes multiple tests for a function that calculates the square of a number, which results in a lot of code.
- The author points out that writing extensive test code manually is inefficient and introduces Pytest as a solution to automate this process.
- Pytest is a third-party library that simplifies writing and running tests by adopting conventions that reduce the amount of code needed.
- The author demonstrates how to use Pytest by rewriting the tests in a more concise manner, reducing the code from 31 lines to 8 lines.
- Pytest automates the process of running tests and provides feedback on which tests pass or fail, making it easier to identify and fix bugs.
- The author explains how to interpret Pytest's output, which indicates whether tests pass or fail and provides clues about the nature of any errors.
- The text also touches on the importance of unit testing, which involves testing individual functions or units of a program.
- The author addresses a question about handling different types of user input, such as strings or floats, and suggests that functions should be designed to handle such cases.
- The author compares Pytest to CS50's Check50 tool, noting that both serve similar purposes but are used in different contexts.
- The text concludes with a discussion on improving test design by automating repetitive tests using loops and other Python structures.
Improving test design enhances debugging
- The speaker initially has five separate tests but only sees the output of the first one due to a failing assertion in the first test. This prevents the other tests from running.
- To improve debugging, the speaker suggests breaking down the tests into different categories, such as testing positive numbers, negative numbers, and zero separately. This approach provides more clues when something goes wrong.
- The speaker demonstrates renaming the function to test_positive and creating separate functions for test_negative and test_zero. This allows all tests to run even if one fails, providing multiple clues for debugging.
- After running the tests with the new structure, the speaker finds that two tests fail and one passes. This helps identify the source of the bug more effectively.
- The speaker fixes the bug in the code (changing addition to multiplication) and reruns the tests, which all pass, indicating the bug is resolved.
- The speaker emphasizes the importance of writing comprehensive tests to catch potential bugs and suggests testing with various inputs, including larger numbers and different data types.
- A question is raised about testing user input and whether to use separate files for different types of tests. The speaker explains that it is possible to use separate files or keep them in one file for convenience.
- Another question addresses the use of the assert keyword and assertion errors. The speaker explains that assert raises an assertion error when the Boolean expression is false, and pytest handles these exceptions to provide standard output.
- The speaker demonstrates adding a test for a type error by using pytest's raises function to check if passing a string to the square function raises a type error.
- The speaker discusses testing code that expects strings as input, using the example of a hello function that prints a greeting. They explain how to import and test this function, focusing on the module rather than user input.
Testing functions effectively
- The text discusses the importance of testing functions in programming, specifically in Python.
- It begins by demonstrating a simple function, 'hello', which prints a greeting based on user input.
- The text explains the difference between functions that return values and those that have side effects, like printing to the screen.
- It highlights the importance of designing functions to return values rather than having side effects to make them more testable.
- The text shows how to modify the 'hello' function to return a string instead of printing it, making it easier to test.
- It introduces the concept of using assert statements to test the return values of functions.
- The text emphasizes the importance of keeping tests simple and small to avoid the need for testing the tests themselves.
- It also covers organizing tests into multiple files and folders, and how to use the 'pytest' framework to run these tests.
- The text concludes by discussing the benefits of automated testing, especially when collaborating with others.
Python file handling and list operations
- The text begins by explaining how to create an empty list in Python and add user-provided names to it using a loop. The loop runs three times, prompting the user for a name each time and appending it to the list using the append method.
- The text discusses the use of an underscore (_) as a variable in loops when the variable is not used later in the code. This is a Pythonic convention to indicate that the loop variable is not important.
- The text then explains how to simplify the code by directly appending the return value of the input function to the list, eliminating the need for an intermediate variable.
- The text describes how to sort the list of names alphabetically using the sorted function and then print each name in the sorted list using a for loop and an f-string for formatting.
- The text introduces the concept of file I/O (input/output) in Python, explaining how to save data to a file so that it persists between program runs. This is compared to saving data in applications on a phone or computer.
- The text explains how to use the open function to open a file for writing. The 'w' mode is used to write to the file, and if the file does not exist, it is created. The file handle returned by open is used to write data to the file and then close it.
- The text demonstrates running the program multiple times to write different names to the file. However, it points out that using 'w' mode overwrites the file each time, resulting in only the last name being saved.
- The text suggests using the 'a' mode (append) instead of 'w' mode to add new names to the file without overwriting the existing content. This ensures that all names are saved in the file.
- The text highlights the importance of adding a newline character ('\n') when writing names to the file to ensure each name appears on a new line. This makes the file content more readable.
- The text introduces the 'with' statement in Python, which is a more Pythonic way to handle files. It ensures that the file is automatically closed after the block of code is executed, reducing the risk of file corruption or data loss.
Python file handling and sorting
- The 'with' statement in Python ensures that files are automatically closed after their block of code is executed, preventing potential errors from forgetting to close files.
- To read names from a file, you can open the file in read mode ('r') and use the 'readlines' method to read all lines into a list. This method returns each line as an element in the list.
- A 'for' loop can be used to iterate over each line in the list and print it. However, this may result in extra new lines due to the newline characters in the file and the 'print' function adding its own newline.
- To remove the extra new lines, you can use the 'rstrip' method to strip the newline character from each line before printing.
- Instead of reading all lines first and then iterating over them, you can directly iterate over the file object in a 'for' loop, which reads one line at a time. This approach is more efficient and compact.
- If you need to sort the lines before printing, you should first read all lines into a list, sort the list, and then iterate over the sorted list to print each line.
- The 'sorted' function in Python can be used to sort the list of lines. You can pass the 'reverse=True' argument to sort the lines in descending order instead of ascending order.
- Combining file reading, sorting, and printing into a single, efficient process is a common technique in Python programming. This involves reading the file, processing the data (e.g., sorting), and then outputting the results.
Python file handling and CSV parsing
- The discussion begins with a demonstration of running a Python script to sort names, emphasizing the importance of consulting documentation for problem-solving.
- A question is raised about limiting the number of names in a file and finding specific names, which leads to a discussion on how to implement such features using Python code.
- The instructor explains how to read all lines from a file, strip unnecessary characters, and why certain design choices are made, such as using R strip to remove whitespace.
- The concept of storing additional information, like a student's house at Hogwarts, is introduced, leading to the idea of using a CSV file instead of a plain text file for better organization.
- CSV (Comma Separated Values) files are explained as a common format for storing related data, and the instructor demonstrates how to create and write to a CSV file in Python.
- The process of reading from a CSV file is detailed, including how to split lines into individual values using the split function and how to handle these values in Python.
- A question about editing specific lines in a file is addressed, explaining that while it's possible, it's often easier to read the entire file, make changes in memory, and then write the file back.
- The instructor introduces a more readable way to handle CSV data by unpacking values directly into variables, improving code clarity and maintainability.
Python sorting with dictionaries
- The text explains how to make Python code more readable by using variables for names and houses instead of hardcoding them. This makes the code functionally the same but easier to understand.
- It demonstrates how to sort a list of students by their names using Python. Initially, the names and houses are read from a CSV file and stored in a list. The list is then sorted and printed.
- The text introduces the concept of using dictionaries to store student information. Each student is represented by a dictionary with keys for 'name' and 'house'. This allows for more organized data management.
- A method for sorting a list of dictionaries by a specific key is explained. A custom function is defined to extract the key (e.g., name or house) from each dictionary, and this function is used as a parameter in the sorted function.
- The text highlights the flexibility of sorting by different keys (e.g., name or house) and in different orders (e.g., ascending or descending). This is achieved by modifying the custom function and the parameters of the sorted function.
- The importance of using dictionaries for more complex data structures is emphasized. This approach allows for better data manipulation and sorting capabilities compared to using simple lists.
- The text concludes with a discussion on the benefits of using libraries and other techniques to automate sorting and other tasks, making the code more efficient and easier to manage.
Using Python's CSV library for efficient data handling
- The text discusses the representation of students using dictionaries in Python, where each student has a name and a house. The goal is to maintain this association using a list of key-value pairs.
- The sorted function in Python is used to sort a list of dictionaries based on the student's name. The get_name function is defined to return the student's name, which is then used by the sorted function to determine the order.
- Instead of defining a separate get_name function, the text introduces the concept of a lambda function, an anonymous function that can be used in place of a named function. The lambda function is used to return the student's name directly within the sorted function.
- The text explains how to handle CSV files in Python, particularly when the data contains commas that can interfere with the CSV format. It suggests using the CSV library in Python to handle these cases more efficiently.
- The CSV library's reader function is introduced, which reads a CSV file and handles the parsing of commas and quotes. This allows for more robust and error-free reading of CSV files.
- The text demonstrates how to use the CSV library to read a CSV file, create dictionaries for each student, and handle cases where the data contains commas within the values.
- The text concludes with an example of modifying the CSV file to contain students' homes instead of houses and updating the code to handle this new data. The CSV library is used to read the updated file and sort the students by their names.
Reading and writing CSV files with Python
- The text discusses the feasibility of making a file both readable and writable simultaneously. It explains that while it is possible, it requires keeping track of the file's position, similar to how cassette tapes work sequentially.
- The recommended approach for modifying files is to read the entire file and then write back the changes, especially for large files where piecemeal changes can be time-consuming.
- The text introduces the concept of CSV (Comma-Separated Values) files and how to read and write them using Python. It explains that CSV files often have a header row that contains the names of the columns, which helps in identifying the data.
- Using a CSV reader, one can read the file line by line, treating each line as a list of columns. However, a more flexible approach is to use a CSV dictionary reader, which treats each line as a dictionary with column names as keys.
- The text provides an example of how to use a CSV dictionary reader to read a CSV file. It shows how to access the values of specific columns by their names, making the code more robust against changes in the file's structure.
- The importance of the newline character in CSV files is discussed. It is a convention that separates data lines, making it easier to read and extract data.
- The text also covers how to write to a CSV file using Python. It explains how to open a file in append mode and use a CSV writer to add new rows to the file.
- An example is provided to demonstrate writing to a CSV file. The example shows how to prompt the user for input and write the data to the file, handling special characters like commas in the input.
- The text introduces the CSV dictionary writer, which allows writing rows as dictionaries. This method is more flexible and can handle changes in the file's structure without breaking the code.
- The text concludes with an example of using a CSV dictionary writer to write data to a CSV file. It shows how to specify the field names and write the data as dictionaries, making the code more maintainable.
Python CSV handling and image manipulation
- The text discusses handling CSV files in Python, focusing on reading and writing using dictionary writers and readers. It explains that dictionary writers allow for flexible ordering of keys and values, ensuring correct column placement through field names.
- A question is raised about the use of single versus double quotes in Python. The response clarifies that either can be used, but consistency is key. It also notes that alternating quotes may be necessary when dealing with nested quotes, such as within f-strings.
- Another question addresses the use of multiple CSV files in a program. The response confirms that multiple CSV files can be used and highlights the importance of using a good library to avoid errors. It also mentions that human errors or external tools like Excel can cause issues.
- The text transitions to discussing file formats, noting that CSVs are just one way to store data. It introduces the concept of binary files, which store data in zeros and ones, and mentions the Pillow library for handling image files in Python.
- A demonstration is provided on creating an animated GIF using the Pillow library. The process involves importing necessary libraries, opening image files, and saving them as an animated GIF. The example uses images from the Scratch programming language to create the animation.
- The code example includes handling command line arguments to specify image files, using a loop to open and store images, and saving the first image with additional frames to create the animation. The importance of specifying the correct command line arguments and handling slices in Python is emphasized.
Python file I/O and regex basics
- The text begins with an example of using Python to manipulate image files. The author demonstrates how to read and write both textual and binary files using the Pillow library. This includes creating a GIF from multiple images and looping it infinitely.
- The author transitions to discussing regular expressions (regex), which are patterns used to match data. Regex is useful for validating user input, such as email addresses, to ensure they meet specific criteria.
- An example is provided where the author writes a Python script to validate email addresses. Initially, the script checks for the presence of an '@' symbol to determine if the input is a valid email address.
- The script is then improved to also check for a dot ('.') in the domain part of the email address. This is done by splitting the email string at the '@' symbol and checking both the username and domain parts.
- Further refinement includes ensuring the domain ends with '.edu', making the validation more specific to educational email addresses. The author explains how to use Python's string methods like 'split' and 'strip' to manipulate and validate the input.
- The author introduces the concept of using the 're' library in Python for more complex pattern matching. This library allows for defining and checking patterns more efficiently than using multiple string methods.
- The text concludes with a brief mention of the capabilities of the 're' library, including validating, replacing, and extracting data based on defined patterns.
Using re.search for email validation
- The text discusses the use of the re.search function from the re module in Python for validating email addresses. The re.search function allows you to search for a pattern within a string, which is useful for tasks like email validation.
- Initially, the text explains how to import the re module and use the re.search function to check if an email address contains an '@' symbol. This basic check is not sufficient for proper email validation as it would accept invalid emails like just an '@' symbol.
- The text then introduces the concept of regular expressions (regex) and how they can be used to create more complex patterns for validation. It explains various regex symbols such as '.', '*', '+', '?', and '{}' which help in defining patterns more precisely.
- The text provides an example of improving the email validation by using the regex pattern '.*@.*' to ensure there is something before and after the '@' symbol. However, this pattern is still not perfect as it would accept invalid emails like 'malan@'.
- To further refine the validation, the text suggests using the '+' symbol instead of '*' in the regex pattern, changing it to '.+@.+'. This ensures that there is at least one character before and after the '@' symbol, making the validation more accurate.
- The text also discusses the concept of finite state machines (FSM) and how they are used by the re.search function to process the input string and match it against the regex pattern. It explains how the FSM transitions between states based on the characters in the input string.
- Finally, the text emphasizes that there are multiple ways to solve the same problem in programming, and different regex patterns can be used to achieve similar results. It encourages understanding the underlying concepts to choose the most appropriate solution for a given problem.
Validating email with regex in Python
- The text discusses how to validate email addresses using regular expressions in Python. It starts by explaining the basic structure of an email address and how to consume characters using regex patterns.
- The initial regex pattern used is dot plus (.+), which matches one or more characters. This pattern is used to ensure that there is at least one character after the '@' sign in the email address.
- The text then highlights a problem with the initial regex pattern: it can match any character, not just a literal dot ('.'). This means that invalid email addresses like 'malan@harvard?edu' would be considered valid.
- To fix this issue, the text introduces the concept of escape characters. By using a backslash (\) before the dot, the regex pattern is modified to match a literal dot. This ensures that only email addresses ending with '.edu' are considered valid.
- The text also explains the importance of using raw strings in Python when working with regex patterns. By prefixing the regex pattern with an 'r', Python is instructed to treat the string as a raw string, preventing any misinterpretation of escape characters.
- The text then demonstrates how to further refine the regex pattern to ensure that the email address starts with a valid username and ends with '.edu'. This is done by using the caret (^) and dollar sign ($) symbols to match the start and end of the string, respectively.
- The text concludes by discussing the importance of precise input validation, especially in contexts like Google Forms or Office 365, where users might input full sentences or incorrect data. Precise regex patterns help ensure that only valid email addresses are accepted.
Regular expressions for email validation
- The dollar sign in regular expressions does not denote currency but matches the end of a string. To match a literal dollar sign, use a backslash before it.
- The dot (.) in regular expressions matches any character, and the plus (+) means one or more of the preceding element. Dot plus (.+) means one or more of any character.
- Using dot star (.*) means zero or more of any character, which can be used to match any string of characters.
- Square brackets in regular expressions define a set of characters to match. A caret (^) inside square brackets negates the set, meaning it matches any character except those listed.
- To restrict characters in an email validation, use square brackets with ranges (e.g., a-z, A-Z, 0-9) and specific characters like underscores.
- The regular expression for email validation must ensure only one at sign (@) is present and that it is surrounded by valid characters.
- The regular expression can be further refined to match specific domain names and ensure compliance with email standards.
Regular expressions simplify pattern matching
- The text discusses the use of regular expressions (regex) for pattern matching in strings, particularly for email validation.
- Regular expressions can be cryptic, but they offer shortcuts for common patterns, such as using '\\w' to represent word characters (letters, digits, and underscores).
- The text explains how to replace complex sets of characters with simpler regex patterns, making the expressions more readable and maintainable.
- It introduces various regex patterns like '\\d' for digits, '\\s' for whitespace, and their complements (e.g., '\\D' for non-digits).
- The text covers the use of parentheses and vertical bars in regex to group patterns and specify alternatives (e.g., matching '.com' or '.edu').
- It explains how to handle spaces and other characters in regex by combining patterns or using square brackets to specify character sets.
- The text addresses case sensitivity in regex and how to use flags like 're.IGNORECASE' to make pattern matching case-insensitive.
- It provides examples of how to validate email addresses using regex, including handling multiple dots in domain names and ensuring the pattern matches the entire string.
Optional subdomain handling in regex
- The speaker discusses modifying a regular expression to optionally support subdomains in email validation.
- Initially, the speaker adds a sequence to the regex to allow for subdomains, but this breaks the validation for emails without subdomains.
- The solution proposed is to use a question mark to make the subdomain part of the regex optional, allowing the regex to match both with and without subdomains.
- The speaker explains the importance of grouping parts of the regex with parentheses to ensure the question mark applies to the entire subdomain sequence.
- An example is given where the regex is tested with and without subdomains, showing that the modification works as intended.
- The speaker also touches on the use of other regex symbols like the asterisk (*) for zero or more repetitions and the vertical bar (|) for alternation.
- The discussion includes a brief mention of using libraries for complex regex patterns, such as those for validating email addresses, to avoid errors and save time.
- The speaker transitions to a new problem of reformatting user input, specifically names, to ensure consistent data storage.
Python input cleanup using regex
- The speaker discusses the challenge of cleaning up user input, specifically names formatted with commas, which is common in the U.S. The goal is to reformat names from 'Last, First' to 'First Last.'.
- The speaker suggests using Python to programmatically clean up the input. They propose checking if a comma is present in the name and then splitting the name into 'last' and 'first' variables based on the comma and space. The name is then reformatted to 'First Last.'.
- The speaker demonstrates running the Python script and shows how it correctly reformats 'Malin, David' to 'David Malin.'.
- The speaker identifies potential issues with the initial approach, such as handling names with suffixes (e.g., 'Jr.') or names without a space after the comma. They demonstrate how these cases can break the code, resulting in errors or incorrect formatting.
- To address these issues, the speaker introduces regular expressions (regex) to make the space after the comma optional. They explain how to use the 're' library in Python to search for patterns and capture groups of characters using parentheses.
- The speaker modifies the code to use 're.search' with a regex pattern that captures the last and first names, even if the space after the comma is missing. They explain how to access the captured groups using 'matches.group(1)' and 'matches.group(2).'.
- The speaker demonstrates the updated code, showing that it can handle both 'Malin, David' and 'Malin, David' without a space, correctly reformatting them to 'David Malin.'.
- The speaker further refines the code by simplifying the assignment of the first and last names directly within the reformatting step, making the code more concise and readable.
- The speaker addresses a question about why the group indices start at 1 instead of 0, explaining that in the context of 're.search,' the 0th index is reserved for the entire match, while subsequent indices correspond to the captured groups.
- The speaker concludes by running the script again to ensure it works correctly with various inputs, demonstrating the robustness of the final solution.
Python regular expressions and walrus operator
- David Malan demonstrates handling different amounts of whitespace in strings using Python's regular expressions.
- He explains how to use the re.search function with capturing parentheses to clean up and standardize user input.
- Malan introduces the walrus operator (:=) in Python, which allows assignment and a boolean check in a single line.
- He shows how to extract specific information from user input, such as a Twitter username from a URL, using string methods like replace and remove_prefix.
- Malan emphasizes the importance of being flexible with user input to improve user experience.
- He discusses the limitations of simple string methods and the advantages of using regular expressions for more complex patterns.
Regular expressions for URL parsing
- The text discusses the use of the re.sub function in Python's regular expression library to substitute patterns in strings. The first argument is the pattern to look for, the second is the replacement string, and the third is the string to perform the substitution on. Additional arguments include flags and count, which control the number of substitutions.
- The example provided demonstrates using re.sub to remove 'https://twitter.com' from a URL string. The result is assigned to a variable called 'username', and the final string is printed using an f-string.
- The text highlights potential issues with the initial solution, such as handling different protocols (HTTP vs. HTTPS) and optional subdomains (www). It suggests using regular expression techniques like the caret symbol (^) to match the beginning of a string and the question mark (?) to make parts of the pattern optional.
- A specific issue is identified with the dot (.) in 'twitter.com', which needs to be escaped with a backslash (\) to avoid matching any character. The text explains that special symbols in regular expressions must be handled carefully to ensure accurate matching.
- The text proposes refining the regular expression to handle both 'http' and 'https' protocols by using 'http' followed by an optional 's' (http?s). It also suggests making 'www.' optional by grouping it with parentheses and following it with a question mark (www\.)?.
- The text discusses making the entire protocol part (http:// or https://) optional by grouping it and adding a question mark. This approach ensures that the regular expression can match URLs with or without the protocol specified.
- The text advises against writing complex regular expressions all at once. Instead, it recommends building them incrementally, testing each step to ensure correctness. This method helps in understanding and debugging the regular expression more effectively.
- The text concludes by suggesting a switch from re.sub to re.search for conditional logic. This change allows the program to verify if the pattern matches before performing actions like printing or saving the username. This approach ensures that only valid Twitter URLs are processed.
Extracting Twitter usernames using regex
- The text discusses how to extract Twitter usernames from URLs using Python and regular expressions (regex).
- It begins by explaining the need to capture everything to the right of the 'twitter.com' URL and how to use regex to achieve this.
- The author uses 're.ignorecase' to make the search case-insensitive and 're.search' to find matches.
- Parentheses are used in the regex pattern to capture the username part of the URL.
- The author demonstrates how to handle optional 'www' subdomains and how to adjust the regex pattern to capture the correct group.
- A mistake is identified and corrected by changing 'matches.group(1)' to 'matches.group(2)' due to the optional 'www' subdomain.
- The author introduces the non-capturing group syntax '(?:)' to avoid capturing unnecessary parts of the URL.
- The regex pattern is refined to only allow valid Twitter username characters (letters, numbers, and underscores).
- The author tests the final regex pattern to ensure it correctly extracts the username from various URL formats.
- Additional regex functions like 're.split' and 're.findall' are briefly mentioned as useful tools for more complex string manipulations.
- The text concludes by highlighting the versatility of regex across different programming languages and its applications in data validation, cleaning, and extraction.
Python functions and tuples
- The text demonstrates how to use Python functions to organize code more effectively. It starts with a simple program that prints a formatted string using user input and then refactors the code to use functions for better structure.
- The main function is introduced to encapsulate the program's logic. Instead of directly using input functions, the code assumes the existence of get_name and get_house functions to retrieve user input, making the code more modular.
- The get_name and get_house functions are defined to prompt the user for their name and house, respectively. These functions are simple but serve as abstractions, allowing the main function to focus on higher-level logic.
- The concept of returning multiple values from a function is explored. Initially, the idea of using a dictionary is considered, but the text opts for returning a tuple instead. This approach is simpler and leverages Python's ability to return multiple values as a tuple.
- The main function is updated to call get_student, which returns a tuple containing the user's name and house. The tuple is unpacked into separate variables for printing. This demonstrates how to handle multiple return values in Python.
- The text explains the difference between lists and tuples in Python. Tuples are immutable, meaning their values cannot be changed after creation. This immutability is useful for ensuring data integrity and preventing accidental modifications.
- An example is provided to illustrate the immutability of tuples. The text attempts to change a value in a tuple, which is not allowed, reinforcing the concept of immutability.
- The text concludes by discussing the practical use of tuples in programming. Tuples are recommended when the data should not change, as they help prevent bugs and maintain code correctness.
Tuples immutable, lists mutable, dictionaries semantic
- The text discusses the use of tuples, lists, and dictionaries in Python programming, focusing on their mutability and use cases.
- Tuples are immutable, meaning their contents cannot be changed once created. This is demonstrated by an error when trying to assign a new value to a tuple element.
- Lists are mutable, allowing changes to their contents. The text shows how to convert a tuple to a list to enable modifications.
- Dictionaries are introduced as a more semantic way to store key-value pairs, making the code more readable and less error-prone compared to using numeric indices in tuples or lists.
- The text explains how to create and manipulate dictionaries, including adding key-value pairs and modifying existing values.
- An example is given where a student's house is corrected from Gryffindor to Ravenclaw using a dictionary, demonstrating the practical use of dictionaries in handling more complex data.
- The text also touches on the syntax differences between tuples, lists, and dictionaries, such as using square brackets for lists and curly braces for dictionaries.
- The importance of readability and maintainability in code is emphasized, suggesting that while compact code is possible, readability should not be sacrificed.
Creating custom data types with classes
- The text discusses the limitations of using basic data types like tuples, lists, and dictionaries for complex data structures in Python.
- It introduces the concept of classes as a solution to create custom data types, allowing for more organized and readable code.
- A class is described as a blueprint or mold for creating objects, which are instances of the class with specific attributes and methods.
- The example of creating a 'Student' class is used to illustrate how to define a class and instantiate objects from it.
- Attributes of the 'Student' class, such as 'name' and 'house', are set using dot notation, which is a common practice in object-oriented programming.
- The text explains the process of defining a class with the 'class' keyword and how to use it to create objects with specific attributes.
- It highlights the benefits of using classes, such as better data organization, error checking, and the ability to define custom methods for added functionality.
- The concept of object-oriented programming (OOP) is introduced, emphasizing the creation of objects from classes and the use of instance variables and methods.
Python class initialization
- The __init__ method, also known as the Dunder init method, is an instance method in Python used to initialize objects of a class. It is called automatically when a new object is created.
- The __init__ method takes 'self' as its first parameter, which refers to the current instance of the class. This allows the method to initialize the object's attributes.
- Attributes such as 'self.name' and 'self.house' are instance variables that store data specific to each object. These attributes are set within the __init__ method using the values passed as arguments.
- The process of creating a new object is known as a constructor call. This involves calling the class name as a function and passing the required arguments, which are then used by the __init__ method to initialize the object.
- The __init__ method ensures that each object is initialized with the necessary attributes, similar to how houses built from the same blueprint can be customized with different colors or decorations.
- Python's __init__ method is distinct from constructors in other languages like Java. While Java uses explicit constructors, Python uses the __init__ method to initialize objects after they are created in memory.
- The 'self' parameter is crucial as it provides access to the current object, allowing the __init__ method to store values in the object's instance variables.
- The __init__ method can also include validation to ensure that the object's attributes meet certain criteria, such as checking that a name is not empty or that a house is one of a predefined set of valid values.
- Object-oriented programming (OOP) in Python allows for better organization and encapsulation of related functionality within classes, making code more modular and easier to manage.
Raising custom exceptions
- The text discusses handling errors in Python programming, specifically focusing on raising custom exceptions.
- It begins by explaining the inadequacy of simply printing error messages or using sys.exit to handle errors, as these methods can be too extreme or insufficient.
- The text introduces the concept of raising exceptions using the 'raise' keyword, allowing programmers to signal specific errors and provide explanatory messages.
- An example is given where a 'ValueError' is raised if a user passes an invalid name or house, demonstrating how to provide meaningful error messages.
- The text also covers how to handle these raised exceptions using try and except blocks, allowing for more controlled error management.
- It explains the benefits of using classes over dictionaries for better control and validation of data attributes.
- The text includes a discussion on how to handle optional variables in classes and how to create custom error types.
- Finally, it touches on the use of special methods like '__str__' to customize the string representation of objects, enhancing the readability of printed output.
Python class methods and attributes
- The __str__ method in Python classes is used to return a string representation of an object. It takes one argument, self, which refers to the current object instance. This method is automatically called when an object is printed.
- In the example, the __str__ method is initially set to return a generic string 'a student'. This is later customized to return the student's name and house using an f-string, making the output more informative.
- The __str__ method is different from the __repr__ method. While __str__ is meant for end-users and provides a readable output, __repr__ is intended for developers and includes more detailed information about the object, such as its type.
- The init method (__init__) is used to initialize an object's attributes. In the example, it is modified to include a third attribute, Patronus, in addition to name and house. This attribute is assigned without validation for simplicity.
- Classes in Python can have both attributes (variables) and methods (functions). Methods are defined within the class and take self as their first argument to access the object's attributes and other methods.
- A custom method named charm is created within the Student class. This method returns an emoji based on the student's Patronus. The method uses a match-case structure to return the appropriate emoji or a default value if the Patronus is unknown.
- The charm method is called outside the class using the dot notation (student.charm). This demonstrates how methods can be accessed and used outside their class definition.
- The example also shows how to prompt the user for input and pass these inputs as arguments to create an instance of the Student class. The inputs include the student's name, house, and Patronus.
- The main function in the example demonstrates how to create a Student object, call its methods, and print the results. It shows the flexibility and power of classes in modeling real-world entities and their behaviors.
Python class properties and decorators
- The text begins by discussing the use of emoji in Python strings, which can be printed like any other character by enclosing them in quotes.
- The author then removes the 'Patronus' code to simplify the example, focusing on core class capabilities. The 'Patronus' attribute and related code are deleted, leaving a 'Student' class with only 'name' and 'house' attributes.
- The 'Student' class has an '__init__' method for initializing objects and a '__str__' method for converting objects to strings. The '__init__' method validates 'name' and 'house' and assigns them to instance variables.
- The author demonstrates that even with validation in the '__init__' method, instance variables can still be modified directly using dot notation, which can bypass validation checks.
- To prevent this, the author introduces Python properties, which are attributes with additional control mechanisms. Properties use getter and setter methods to manage access and modification of instance variables.
- The 'property' decorator is used to define a getter method for the 'house' attribute, which returns the value of 'self._house'. A setter method is also defined to validate and set the 'house' attribute, raising a 'ValueError' if the value is invalid.
- The author explains that using the 'property' decorator and defining getter and setter methods allows for error checking and validation whenever the attribute is accessed or modified, ensuring data integrity.
- The author modifies the '__init__' method to use the setter method for 'house', ensuring that validation occurs both during object creation and when the attribute is modified later.
- The text concludes with a demonstration of the updated 'Student' class, showing that invalid values for 'house' are now correctly rejected, maintaining the integrity of the data.
Python properties ensure controlled attribute access
- The main function attempts to change Harry's house to an invalid value, but Python's property mechanism prevents this by using a setter function that raises a ValueError if the value is not valid.
- The code demonstrates how to protect data by using the init method and setter functions to ensure that only valid values are assigned to attributes.
- The use of getters and setters in Python allows for controlled access to class attributes, ensuring that invalid values cannot be assigned directly.
- Getters in Python take one argument (self), while setters take two arguments (self and the value to be set), allowing for validation before assignment.
- Using self.house in the init method ensures that the setter is called, providing a single point of validation and error checking for the attribute.
- The code also defines a property for the name attribute, with similar getter and setter functions to ensure valid values are assigned.
- Python relies on conventions rather than hard constraints, meaning that while properties can control attribute access, they can be bypassed by directly accessing the underlying instance variable with an underscore prefix.
- The underscore prefix in Python is a convention to indicate that an instance variable is private and should not be accessed directly, but this is not enforced by the language.
- The example highlights the importance of following conventions in Python to ensure code integrity, as the language does not enforce strict access controls.
- The discussion concludes by noting that many built-in Python types, such as int, str, and list, are implemented as classes with methods that provide additional functionality.
Python classes and methods
- The text explains how to iterate over lists and append values using the append method of the list class. It highlights that dictionaries in Python are also classes with methods, and manipulating dictionaries involves using these methods.
- The author demonstrates how to use the type function to determine the data type of a value, such as an integer, string, list, or dictionary. This function is useful for understanding the underlying class of a value.
- The text discusses the convention in Python of using lowercase for built-in data types like int, str, and list, while user-defined classes typically start with an uppercase letter. This distinction helps in identifying built-in versus custom classes.
- The author introduces the concept of class methods, which are associated with the class itself rather than instances of the class. Class methods use the @classmethod decorator and do not have access to instance-specific data (self).
- An example is provided using a Sorting Hat class inspired by Harry Potter. The class includes an __init__ method to initialize a list of houses and a sort method to randomly assign a house to a student. This example illustrates the use of instance variables and methods within a class.
- The text emphasizes the importance of organizing code by placing constants and lists at the top of the class for clarity and maintainability. This practice ensures that any changes to these values are easily identifiable and manageable.
Python class methods and variables
- The discussion begins with the importance of not tucking away lists in functions if they are to be used in multiple functions. Instead, store them in the object itself using self.houses.
- An error occurs when trying to assign Harry to a house because the random library was not imported. The solution is to import the random library at the top of the file.
- The program is tested multiple times, showing that Harry is assigned to different houses randomly, demonstrating the use of the random library.
- The concept of classes is introduced, explaining that a class can represent real-world or fantasy entities. Examples include a Sorting Hat, which is a fantasy entity, and students, which are real-world entities.
- The difference between instance methods and class methods is explained. Instance methods are tied to specific objects, while class methods are tied to the class itself and do not require instantiation of objects.
- Class variables are introduced as variables that exist within the class itself and are shared among all objects of that class. This is contrasted with instance variables, which are specific to each object.
- The code is refactored to use class methods and class variables, eliminating the need to instantiate multiple Sorting Hat objects. This is done using the @classmethod decorator and cls as a reference to the class.
- The benefits of using classes for organizing code are discussed. Classes help encapsulate related data and functionality, making the code more manageable, especially as it grows in complexity.
- A comparison is made between using classes and using functions or modules. While simple problems can be solved with functions, more complex problems benefit from the organization provided by classes.
- The discussion concludes with a proposal to apply the same class method concept to another piece of code, emphasizing the flexibility and utility of object-oriented programming.
Class methods enhance code organization
- The speaker begins by simplifying the code to focus on key ideas, removing properties and error checking to highlight the essence of the class, which includes the student's name, house, and printing functionality.
- The speaker points out that having a function like 'get_student' outside the class, which prompts for a student's name and house and then creates a student object, is not wrong but indicates bad design. This separation of related functionality is considered a 'code smell,' suggesting potential future problems.
- To address this, the speaker demonstrates how to move the 'get_student' functionality into the student class itself by defining a class method called 'get.' This method takes the class itself as an argument and returns a new student object, thus encapsulating all student-related functionality within the class.
- The speaker explains the concept of class methods, which allow methods to be called on a class itself without needing to instantiate an object first. This avoids the 'chicken and egg' problem where an object would need to be created to call a method that creates an object.
- The speaker shows how to modify the main function to use the new class method 'get' instead of the standalone 'get_student' function, thus keeping all student-related code within the student class and making the code more organized and easier to read.
- The speaker tests the modified code to ensure it works as expected, demonstrating that the new design maintains functionality while improving code organization.
- The speaker addresses a question about the order of class and function definitions, explaining that Python reads the code top to bottom, so the order does not matter as long as the main function is called at the end.
- The speaker suggests that for better organization, classes could be defined in their own files and imported as needed, making the code more modular and reusable.
- The speaker briefly touches on the concept of static methods and other advanced features of object-oriented programming, indicating that these are additional tools available for organizing and structuring code.
- The speaker introduces the concept of inheritance in object-oriented programming, where classes can inherit attributes and methods from other classes, allowing for hierarchical class design and reducing code redundancy.
Inheritance in Python classes
- The text begins by discussing the creation of a new class called Wizard, which will serve as a base class for both Student and Professor classes. The Wizard class is initialized with a name and includes error checking to ensure a name is provided.
- The Student and Professor classes are then modified to inherit from the Wizard class. This is done by specifying Wizard as the superclass in the class definition. This allows Student and Professor to inherit the functionality of the Wizard class, such as name assignment and error checking.
- To ensure that the initialization method of the Wizard class is called when creating a Student or Professor object, the super() function is used within the __init__ methods of the Student and Professor classes. This calls the __init__ method of the superclass (Wizard) and passes the necessary arguments.
- The text explains the concept of inheritance in object-oriented programming, where a subclass inherits the properties and methods of its superclass. This allows for code reuse and the creation of more organized and modular code.
- The text also touches on the idea of multiple levels of inheritance, where a class can inherit from a superclass, which in turn inherits from another superclass. This creates a hierarchy of classes, allowing for the inheritance of functionality from multiple levels.
- The concept of multiple inheritance, where a class can inherit from more than one superclass, is briefly mentioned. This allows a class to inherit functionality from multiple sources, but it can also introduce complexity and potential conflicts.
- The text provides an example of how these classes can be used to create objects representing students, professors, and generic wizards. It demonstrates how the inherited functionality is utilized when creating these objects.
- The text also discusses the use of exceptions in Python, explaining that exceptions are organized hierarchically, with more specific exceptions inheriting from more general ones. This allows for the handling of exceptions at different levels of specificity.
- The idea of operator overloading is introduced, where operators like + and - can be redefined to perform different operations depending on the context. This allows for more flexible and intuitive code.
- The text concludes with a mention of implementing a new class for a vault in the context of the Harry Potter universe, which will store different types of coins. This serves as a segue into further exploration of object-oriented programming concepts.
Python class for bank vault operations
- The text describes creating a Python class named 'Vault' to represent a bank vault, which can store galleons, sickles, and canuts. The class uses an __init__ method to initialize these values, allowing for optional parameters with default values of zero.
- Instance variables are used to store the values passed during initialization. The text suggests adding error checking and validation but focuses on the basic implementation for simplicity.
- A __str__ method is defined to provide a string representation of the vault's contents, making it easier to print and understand the stored values.
- An example is given where two vaults are created for 'The Potters' and 'The Weasleys' with different amounts of galleons, sickles, and canuts. The __str__ method is used to print the contents of these vaults.
- The text explains how to combine the contents of two vaults by manually adding the galleons, sickles, and canuts from each vault and creating a new vault with the combined values.
- Operator overloading is introduced to simplify the addition of two vaults. The __add__ method is defined to allow the use of the '+' operator to combine the contents of two vaults automatically.
- The __add__ method takes 'self' and 'other' as parameters, representing the two vaults being added. It sums the galleons, sickles, and canuts from both vaults and returns a new vault with the combined values.
- The text demonstrates the use of the overloaded '+' operator to add two vaults and print the combined contents, showing the effectiveness of operator overloading.
- Questions and answers at the end of the text discuss the possibility of adding other operators, such as subtraction, and the limitations of defining new operators in Python.
Comprehensive Python programming course
- The course covers Python programming in depth, focusing on the design and implementation of web applications using frameworks like Django, React, and Bootstrap.
- Key topics include database design, scalability, security, and user experience, along with writing and using APIs, creating interactive UIs, and leveraging cloud services like GitHub and Heroku.
- Students will learn to read, write, test, and debug code, with a focus on functions, variables, conditionals, loops, exceptions, libraries, unit tests, file I/O, regular expressions, and object-oriented programming.
- The course is suitable for students with or without prior programming experience and offers hands-on practice through exercises inspired by real-world programming problems.
- CS50P is a specialized course focusing entirely on Python, unlike CS50x which covers broader computer science topics and multiple programming languages.
- The final lecture emphasizes advanced Python features and encourages students to explore further through Python's extensive documentation and additional resources.
Python global vs local variables
- The text discusses the implementation of deposit and withdraw functions in Python, focusing on handling global and local variables. The initial approach involves defining a global variable 'balance' and modifying it within the functions using shorthand notation for incrementing and decrementing.
- An error occurs when trying to modify the global variable 'balance' within the functions, resulting in an 'UnboundLocalError'. This error indicates that Python cannot modify a global variable from within a function without explicit declaration.
- The text explains that while global variables can be read within functions, they cannot be written to unless explicitly declared as global within the function. This is demonstrated by moving the 'balance' variable inside the main function, which still results in an error due to the variable being local to the main function.
- To resolve the issue, the text introduces the 'global' keyword in Python, which allows functions to modify global variables. By declaring 'balance' as global within the deposit and withdraw functions, the error is resolved, and the functions work as intended.
- The text then transitions to a more advanced solution using object-oriented programming (OOP). It defines a class 'Account' with an instance variable '_balance' and methods for depositing and withdrawing funds. The class encapsulates the balance and provides a cleaner and more scalable approach.
- The OOP approach includes defining properties to control access to the instance variable '_balance'. The 'balance' property allows reading the balance while preventing direct modification, ensuring better encapsulation and data protection.
- The text concludes by comparing the use of global variables and OOP, recommending the latter for better encapsulation and maintainability. It emphasizes using global variables sparingly and highlights the benefits of OOP in managing complex data and functions.
Python constants are convention-based
- In Python, constants are not enforced by the language but are indicated by conventions such as capitalizing variable names.
- A simple program example is provided where a cat meows three times, demonstrating the use of a constant variable to avoid hardcoding values.
- The importance of surfacing constant values to the top of the code is emphasized to make it easier to manage and change them.
- Python's lack of enforced constants is contrasted with other languages that have keywords or mechanisms to prevent changes to constant values.
- Class constants in Python are also convention-based, and an example is given using a class to represent a cat with a default number of meows.
- Python is dynamically typed, meaning it does not require explicit type declarations, which can lead to type errors if not managed properly.
- Type hints can be used in Python to indicate the expected types of variables, but they are not enforced by the language itself.
- The MyPy tool is introduced as a way to check for type correctness in Python code, helping to catch type errors before the code is run.
Type hints improve code reliability
- The speaker discusses the use of type hints in Python to improve code reliability and catch errors before runtime. Type hints help tools like MyPy to identify type mismatches early, preventing potential bugs.
- An example is given where a variable 'number' should be an integer, but the return value of 'input' is a string. By annotating the variable with 'int', MyPy quickly identifies the type mismatch, allowing the programmer to correct it before running the code.
- The speaker demonstrates converting the return value of 'input' to an integer to avoid type errors. This involves using the 'int' function to ensure the variable 'number' is correctly typed.
- The importance of type hints in larger and professional codebases is emphasized. They help in defensive programming by reducing the probability of bugs and ensuring code correctness.
- A hypothetical scenario is presented where a function 'meow' is mistakenly assumed to return a string. The speaker shows how type hints can catch this mistake by indicating that 'meow' actually returns 'None'.
- The speaker explains how to annotate a function's return type using the arrow notation in Python. This helps tools like MyPy to verify that the function's behavior matches its type annotations.
- The speaker modifies the 'meow' function to return a string instead of printing it, demonstrating how type hints guide the correct implementation of functions.
- The concept of operator overloading in Python is briefly touched upon, explaining how multiplication can be used to repeat strings.
- The speaker introduces docstrings, a standardized way of documenting functions in Python. Docstrings are written inside the function using triple quotation marks and provide a formal way to describe the function's behavior.
Documenting Python code with docstrings
- Python uses triple quotes for docstrings to document functions. These docstrings can be automatically extracted to generate documentation like web pages or PDFs.
- Docstrings help standardize information for human programmers, detailing function arguments, return types, and potential exceptions. This makes the code more understandable and usable by others.
- A common convention for documenting arguments in Python is to use a format like 'param n: description' and 'type n: int'. This helps clarify what each argument represents and its expected type.
- If a function can raise an exception, it should be documented. For example, if an argument is not of the expected type, a TypeError might be raised. This proactive documentation helps other programmers understand potential issues.
- The syntax used in the example is reStructuredText, a popular convention in Python for documentation. It is not a Python feature but a third-party convention that helps in generating documentation automatically.
- Docstrings can also include sample inputs and outputs, which can be used by tools to test the code and ensure it behaves as expected. This adds an extra layer of error checking.
- The example transitions to modifying a Python program to take command line arguments using the sys.argv library. This allows the program to accept user input directly from the command line.
- Command line arguments can be standardized using flags like '-n' for specifying the number of times an action should be performed. This makes the program more user-friendly and easier to understand.
- The example demonstrates how to handle different command line arguments and how to provide usage instructions if the input is incorrect. This is a common practice to guide users on how to use the program.
- For more complex command line argument handling, the argparse library is recommended. It simplifies the process of parsing command line arguments and supports more advanced features like handling multiple flags and options.
Using ARG parse for command line arguments
- The text explains how to use the ARG parse library in Python to handle command line arguments automatically, allowing developers to focus on the core logic of their programs.
- It starts by importing the ARG parse library and creating an argument parser object using ARG parse.ArgumentParser(). This object is then configured to recognize specific command line arguments using the add_argument() method.
- The parser.parse_args() method is used to parse the command line arguments, storing the results in an object. This object contains the values of the arguments, regardless of their order.
- The text demonstrates how to iterate over the parsed arguments and use them in the program. It highlights the advantage of ARG parse in handling different orders of arguments and providing default values.
- The text also covers how to add descriptions and help messages to the arguments, making the program more user-friendly. This is done using the description and help parameters in the add_argument() method.
- It shows how to handle errors when invalid arguments are provided, and how ARG parse automatically generates error messages and usage information.
- The text concludes by emphasizing the benefits of using libraries like ARG parse to automate common tasks, allowing developers to focus on more interesting and complex parts of their programs.
Python unpacking lists and dictionaries
- The speaker demonstrates how to use Python to perform arithmetic operations and store the result in a variable. They use a function to calculate the total value of different coins and print the result in a specific unit of measure.
- The speaker explains how to store multiple coin values in a list and pass them to a function using numeric indices. They highlight the limitations of this approach, such as verbosity and potential for errors.
- The concept of unpacking a list in Python is introduced. By using an asterisk (*) before a list variable, the list can be unpacked into individual arguments when passed to a function. This simplifies the code and reduces verbosity.
- The speaker runs a Python script to demonstrate the correct and incorrect ways of passing a list to a function. They show how passing a list directly results in a type error, while unpacking the list with an asterisk works correctly.
- The speaker discusses the limitations of unpacking lists and introduces the concept of unpacking dictionaries using double asterisks (**). This allows passing key-value pairs from a dictionary to a function as named arguments.
- A demonstration is given on how to convert a list of coin values into a dictionary and pass it to a function using double asterisks. The speaker explains how this approach maintains the order and structure of the data.
- The speaker addresses questions about unpacking with different data structures, such as tuples and sets. They clarify that unpacking works with tuples but not with sets due to the lack of order preservation in sets.
- The speaker explains how to handle cases where the dictionary has more key-value pairs than the function expects. They demonstrate how passing additional arguments results in a type error and emphasize the importance of matching the function's expected parameters.
Python function argument flexibility
- The text discusses the use of single and double asterisks in Python functions to handle variable numbers of arguments. Single asterisks (*) are used for positional arguments, while double asterisks (**) are used for keyword arguments.
- Default values for function arguments allow for flexibility in the number of arguments passed. If default values are set, fewer arguments can be passed without causing errors.
- The text explains how to define a function that can take a variable number of positional and keyword arguments using *args and **kwargs. This allows for more dynamic and flexible function definitions.
- An example is provided where a function f is defined to take any number of positional and keyword arguments. The function prints these arguments to demonstrate how they are handled.
- The text also explains how Python's built-in functions, like print, use similar syntax to handle variable numbers of arguments. The print function can take multiple arguments and has default values for separators and end characters.
- The concept of variadic functions is introduced, which are functions that can take a variable number of arguments. This is useful for creating more flexible and reusable code.
- The text concludes with a brief mention of different programming paradigms supported by Python, including procedural, object-oriented, and functional programming. It hints at the power and flexibility of Python in supporting various programming styles.
Python programming with practical examples
- The speaker discusses improving a function named 'yell' to handle multiple words instead of a single phrase. The goal is to pass multiple words as individual elements in a list.
- Initially, the speaker attempts to convert each word to uppercase using a loop and appending the results to a new list. This approach is functional but not optimal.
- The speaker introduces the concept of unpacking syntax in Python, which allows for more elegant handling of lists when printing. By using the unpacking operator '*', the list elements are printed without Python-specific syntax like quotes and brackets.
- To further improve the function, the speaker suggests using the 'map' function. 'Map' applies a given function to all items in a list, making the code more concise and efficient. The 'map' function is an example of functional programming in Python.
- The speaker then demonstrates an even more Pythonic way to achieve the same result using list comprehensions. List comprehensions allow for the creation of lists in a single line of code, making the code more readable and concise.
- The speaker explains how list comprehensions can include conditionals to filter elements. This is shown through an example where only students from Gryffindor are included in a new list.
- The concept of functional programming is revisited with the introduction of the 'filter' function. 'Filter' is used to create a new list based on a condition, similar to list comprehensions but with a functional approach.
Efficient Python coding techniques
- The text begins by discussing how to simplify conditional statements in Python. Instead of using verbose conditionals to return true or false, you can directly return the Boolean expression itself.
- It then introduces the 'filter' function, which is used to create a list of Gryffindor students. The 'filter' function takes a function and a sequence, applying the function to each element in the sequence to determine if it should be included in the final list.
- The text explains how to use the 'sorted' function with a key argument to sort a list of dictionaries. A Lambda function is used to specify the sorting key, which in this case is the student's name.
- Two approaches to filtering and sorting are compared: using list comprehensions with conditionals and using the 'filter' function. Both methods achieve the same result but have different readability and style implications.
- The text addresses a question about code readability and formatting, specifically mentioning the 'black' formatter. It explains that 'black' would reformat code to improve readability, and the author proactively adjusted the code accordingly.
- A question about using Lambda functions directly within 'filter' is answered. The text demonstrates how to replace a named function with a Lambda function directly in the 'filter' call, simplifying the code.
- The concept of dictionary comprehensions is introduced. This allows for the creation of dictionaries in a concise manner, similar to list comprehensions. The text provides an example of building a dictionary of Gryffindor students.
- The text concludes with a demonstration of converting a list of student names into a dictionary where the keys are student names and the values are 'Gryffindor'. This showcases the flexibility and power of comprehensions in Python.
Python enumerate simplifies iteration
- The text discusses how to print a list of student names with their numerical ranks using Python.
- Initially, a for loop with range and length functions is used to print the names with indices starting from 0, which is not user-friendly.
- To make the indices start from 1, a simple arithmetic operation (i + 1) is introduced.
- The text then introduces the Python built-in function 'enumerate' which simplifies the process by providing both the index and the value simultaneously.
- Using 'enumerate', the code becomes more succinct and readable, eliminating the need for range and length functions.
- The text transitions to a new example involving a program that prints a specified number of sheep to help users fall asleep.
- The initial implementation uses a for loop to print sheep emojis, but it is refined by defining a main function and a helper function 'sheep' to generate the sheep strings.
- The text highlights the problem of running out of memory when generating a large number of sheep, leading to the introduction of Python generators.
- Generators, using the 'yield' keyword, allow the function to return data incrementally, preventing memory overload.
- The text concludes by emphasizing the importance of modularizing code and using best practices like unit testing, even when dealing with large data sets.
Python yield for memory efficiency
- The speaker discusses a problem with a program that uses too much memory and CPU when trying to handle large numbers of sheep in a list.
- To solve this, the speaker suggests using the 'yield' keyword in Python, which allows the function to return one value at a time instead of building a massive list all at once.
- The 'yield' keyword helps in generating data incrementally, which is more memory efficient, especially for large datasets.
- The speaker demonstrates how to modify the code to use 'yield' instead of 'return', allowing the program to handle large numbers of sheep without crashing.
- The concept of 'generators' and 'iterators' is introduced, explaining that 'yield' returns an iterator that can be stepped over one element at a time in a loop.
- A question is raised about how 'yield' works under the hood, and it is explained that the generator retains state and returns values asynchronously, without running the entire loop at once.
- The speaker emphasizes that using 'yield' reduces memory usage significantly, as it only returns one string of sheep at a time instead of all at once.
- The session concludes with a summary of the course, highlighting the various programming concepts covered, such as functions, variables, conditionals, loops, exceptions, libraries, unit tests, file I/O, regular expressions, and object-oriented programming.
- The speaker encourages students to apply their skills to real-world projects and continue learning by asking questions and seeking out resources.
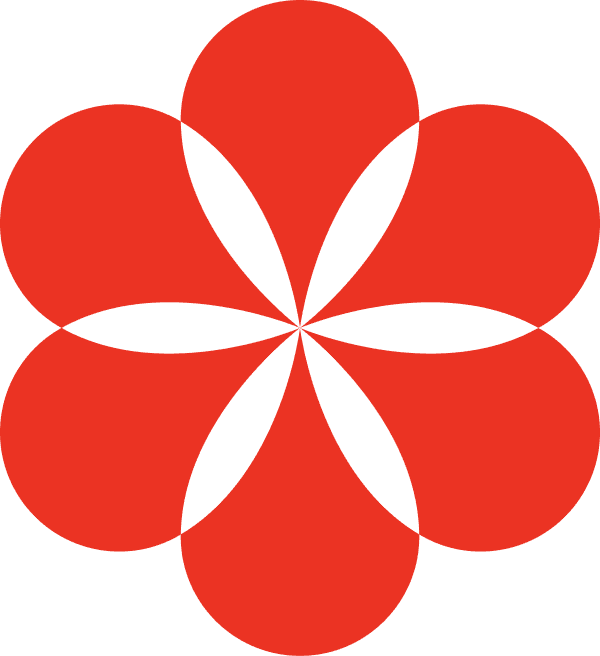
Turn any content into your knowledge base with AI
Supamind transforms any webpage or YouTube video into a knowledge base with AI-powered notes, summaries, mindmaps, and interactive chat for deeper insights.