Mastering JavaScript: From Basics to Advanced
InfoThis is a summary of the following YouTube video:

JavaScript Tutorial Full Course - Beginner to Pro (2024)
SuperSimpleDev
May 9, 2024
ยท
Education
Intro
- The course aims to teach how to build complex websites using JavaScript, starting from a beginner level to a professional level.
- By the end of the course, students will build an interactive multi-page website similar to Amazon.com, where users can add products to their cart, create orders, and track orders.
- Smaller projects like a rock-paper-scissors game, a to-do list, and a calculator will also be built throughout the course.
- No prior coding or technical experience is required as the course covers everything needed to learn JavaScript from the basics.
- The course will cover major JavaScript features and how to use JavaScript with HTML and CSS.
- Advanced features such as object-oriented programming, backend development, callbacks, promises, and async/await will be taught and used in the Amazon project.
- The course includes over 250 exercises to practice the skills learned in each lesson.
- Students can find the different lessons below the video and adjust the video speed as needed.
1 JavaScript Basics
- The course begins by addressing technical issues and ensuring students know where to find solutions in the comments section below the video.
- The course is conducted on Windows, but the steps are applicable to Mac users as well.
- JavaScript is introduced as a technology used to create interactive websites, alongside HTML and CSS.
- HTML is responsible for creating the content of a website, such as buttons, text, and images.
- CSS is used to style the website, making it visually appealing.
- JavaScript adds interactivity to the website, enabling actions like adding products to a cart or creating orders when buttons are clicked.
- The course focuses on JavaScript, with references to separate courses for HTML and CSS for those interested in learning more about them.
- Students are instructed to install a web browser, with Google Chrome recommended for web development.
- The process of downloading and installing Google Chrome is explained for both Windows and Mac users.
- JavaScript is described as a way to give instructions to a computer, which the computer then follows.
- Students are guided to open Google Chrome, navigate to a specific URL, and access the console to start writing JavaScript code.
- The first JavaScript instruction taught is the 'alert' function, which creates a popup with a specified message.
- The importance of typing code correctly is emphasized, as incorrect syntax will result in errors.
- Students practice creating popups with different messages using the 'alert' function.
- The concept of 'running the code' is introduced, which refers to the computer executing the given instructions.
- Different programming languages are mentioned, with JavaScript being the focus of this course.
- Students learn to perform basic math operations in the console, such as addition and subtraction.
- An example is provided to demonstrate how JavaScript can modify the content of a webpage.
- The term 'syntax' is introduced, referring to the rules that must be followed when writing code.
- The importance of following syntax rules exactly is highlighted, as failure to do so will result in errors.
- Students are reassured that they do not need to memorize all syntax rules immediately, as they will learn them gradually throughout the course.
- The lesson concludes with a summary of the key points covered, including the basics of JavaScript, running code, and understanding syntax.
- Students are encouraged to practice what they have learned through exercises provided in the video description.
2 Numbers and Math
- The lesson focuses on numbers and math in JavaScript, starting with basic arithmetic operations like addition, subtraction, multiplication, and division.
- The syntax for math in JavaScript is straightforward, resembling normal math expressions. JavaScript can handle both integer and decimal (floating point) numbers.
- Practical examples include calculating the cost of products in a shopping cart, including shipping and taxes, using JavaScript in the console.
- The order of operations (operator precedence) in JavaScript follows standard math rules: multiplication and division are performed before addition and subtraction. Brackets can be used to control the order of operations.
- JavaScript can sometimes produce inaccurate results with floating point numbers due to how computers store these numbers. This issue is common across many programming languages.
- To avoid inaccuracies when dealing with money, it's best to perform calculations in cents and then convert back to dollars.
- Rounding numbers in JavaScript can be done using the Math.round function, which rounds a number to the nearest integer.
- The lesson also covers how to use Google to find JavaScript code snippets and understand new features, emphasizing that it's okay not to understand all the code initially.
3 Strings
- The lesson begins by reviewing the previous topic on numbers and math, and introduces the new topic of working with text using strings.
- Students are instructed to open the final project on the checkout page and ensure the console is open for practice.
- A string in JavaScript represents text and is created by enclosing text in single quotes. For example, typing 'hello' in the console creates a string with the text 'hello'.
- The lesson revisits the 'alert' function from the first lesson, explaining that the text inside the alert is a string.
- Syntax rules for strings are explained: text is enclosed in single quotes, and strings can be concatenated (combined) using the '+' operator.
- The concept of type coercion is introduced, where JavaScript automatically converts numbers to strings when they are added together.
- Practical examples are provided, such as creating text for the final project by combining strings and numbers, and using brackets to ensure correct order of operations in calculations.
- The lesson explains the importance of calculating in cents to avoid inaccuracies with floating-point arithmetic when dealing with money.
- Students are guided through creating a popup that displays a line of text using the 'alert' function and the strings they created.
- Three ways to create strings in JavaScript are introduced: single quotes, double quotes, and backticks (template strings).
- The lesson discusses the use of escape characters to handle special characters within strings, such as single quotes, double quotes, and new lines.
- Template strings are highlighted for their special features, including interpolation (inserting values directly into strings) and multi-line strings.
- The lesson concludes with a recommendation to use single quotes by default, and template strings when needing to insert values or create multi-line strings.
4 HTML CSS Review, console.log
- The lesson begins with a review of basic JavaScript features like numbers and strings, and introduces the integration of HTML, CSS, and JavaScript to build a full website.
- HTML (HyperText Markup Language) is used to create the content of a website, such as buttons and text. CSS (Cascading Style Sheets) changes the appearance of the website, while JavaScript makes the website interactive.
- To write HTML and CSS code, a code editor like Visual Studio Code (VS Code) is needed. Instructions for installing VS Code are provided.
- HTML code is written inside a file using a code editor. The lesson demonstrates creating a new folder, opening it in VS Code, and creating an HTML file named 'website.html'.
- Basic HTML syntax is reviewed, including creating elements like buttons and paragraphs using tags. The concept of opening and closing tags is explained.
- The lesson covers nesting elements, where one element is placed inside another, and the handling of multiple spaces and new lines in HTML, which are combined into a single space on the webpage.
- CSS syntax is introduced, including selectors, properties, and values. The lesson demonstrates changing the appearance of elements, such as setting the background color and text color of buttons.
- HTML attributes are reviewed, which change the behavior of elements. The 'class' attribute is introduced to style specific elements differently using CSS.
- The HTML structure is explained, including the 'doctype', 'html', 'head', and 'body' elements. The importance of following this structure for access to more HTML features is emphasized.
- The lesson demonstrates setting up VS Code for better readability, including adjusting indentations and enabling line wrapping.
- JavaScript can be run inside an HTML file using the 'script' element or the 'onclick' attribute. The lesson shows how to create alerts and handle button clicks with JavaScript.
- Comments in JavaScript, HTML, and CSS are introduced, which are ignored by the computer and used for providing information or disabling code without deleting it.
- The 'console.log' function in JavaScript is explained, which displays the result of code in the console, useful for debugging and checking outputs.
5 Variables
- The lesson begins with an introduction to JavaScript variables, explaining that they are used to store values like numbers or strings for later use.
- A step-by-step guide is provided to create a new HTML file named '05-variables.html' and copy the content from 'website.html' to this new file, preparing it for the lesson on variables.
- The concept of variables is introduced by creating a variable named 'variable1' using the 'let' keyword and assigning it the value of 3. The value is then displayed in the console using 'console.log'.
- An example is given to create another variable named 'calculation' that stores the result of 2 + 2. This value is also displayed in the console.
- The lesson explains how to use variables in calculations and how to reassign values to variables. For instance, 'variable1' is reassigned the value of 5, and this change is reflected in the console output.
- The syntax rules for variables are discussed, including the use of the 'let' keyword, naming conventions, and the importance of semicolons to end instructions.
- A project is introduced to create a simple cart quantity feature. The project involves creating buttons in HTML and using JavaScript to update and display the cart quantity in the console.
- The lesson covers how to make the buttons interactive using the 'onclick' attribute and how to update the cart quantity variable when different buttons are clicked.
- Shortcuts for reassigning variables are introduced, such as using '+=', and the lesson explains the importance of using meaningful variable names following camelCase convention.
- The lesson concludes with an explanation of the three ways to create variables in JavaScript: 'let', 'const', and 'var', and the best practices for using each. It also touches on using 'typeof' to check the type of value stored in a variable.
6 Booleans and If-Statements
- The lesson begins with creating a new HTML file named '06-booleans.html' and copying the code from 'variables.html' into this new file. The JavaScript code is removed, but the script element is kept.
- Booleans are introduced as a type of value in JavaScript with only two possible values: true and false. These values represent whether something is true or false. Examples are given using comparison operators like '<' and '>' to show how booleans work.
- The syntax rules for booleans are explained, emphasizing that booleans are not surrounded by quotes. The 'typeof' operator is used to differentiate between strings and booleans.
- Comparison operators are discussed, including '<', '>', '<=', '>=', '===', and '!=='. The difference between '==' and '===' is highlighted, with '===' being preferred to avoid type conversion.
- The lesson introduces if-statements, which allow for conditional execution of code. The basic structure of if-statements is explained, including the use of 'else' for alternative code execution.
- A practical example is provided to check if a person is old enough to drive using if-statements. The concept of 'else if' is introduced to handle multiple conditions.
- The lesson explains how to combine if-statements with variables, using 'const' and 'let' to store values and reuse them in conditions.
- A project is introduced to build a simple rock-paper-scissors game. The steps include creating HTML elements, generating a random move for the computer using 'Math.random()', and comparing moves using if-statements.
- Logical operators ('&&', '||', '!') are introduced to combine boolean values. The '&&' operator checks if both sides are true, '||' checks if at least one side is true, and '!' flips the boolean value.
- The concept of scope is explained, showing how variables created inside if-statements are limited to that scope. The lesson advises creating variables outside if-statements to access them globally.
- The lesson covers truthy and falsy values, explaining that non-boolean values can behave like booleans in if-statements. A list of falsy values is provided, including false, 0, '', NaN, undefined, and null.
- Shortcuts for if-statements are introduced, including the ternary operator (a ? b : c), the guard operator (a && b), and the default operator (a || b). These shortcuts provide more concise ways to write conditional logic.
- The lesson concludes with a summary of key concepts: booleans, if-statements, comparison and logical operators, algorithms, truthy and falsy values, and shortcuts for if-statements. Exercises are provided for practice.
7 Functions
- The lesson focuses on learning JavaScript functions and applying them to improve a rock-paper-scissors project.
- First, a new file named '07-functions.html' is created by copying the content from 'booleans.html' and modifying it for the lesson.
- Functions in JavaScript allow code reuse by defining a block of code that can be executed multiple times by calling the function name with parentheses.
- The syntax for creating a function includes the 'function' keyword, a function name, parentheses, and curly brackets containing the function body.
- Function names follow the same rules as variable names and should use camelCase.
- To execute a function, it must be called by typing its name followed by parentheses, a process known as calling or executing the function.
- Functions help in reducing code duplication and make the code easier to update by centralizing repeated code blocks.
- The concept of scope is introduced, explaining that variables defined inside a function are not accessible outside of it, which can be managed by using global variables or return statements.
- Return statements allow functions to output a value, which can be used or stored in a variable outside the function.
- Parameters are introduced as a way to pass values into functions, making them more flexible and reusable for different inputs.
- The lesson demonstrates improving the rock-paper-scissors project by creating functions to handle repeated code blocks and using parameters to handle different player moves.
- The importance of tracing code execution step-by-step is emphasized to understand how functions and parameters work together.
- The lesson concludes with a summary of the benefits of using functions, including code reuse, reduced duplication, and easier updates.
8 Objects
- The lesson introduces JavaScript objects, a type of value that groups multiple values together.
- A new file named '08-objects.html' is created, and the code from 'functions.html' is copied into it.
- The script element is prepared by changing the title to 'objects' and removing existing code.
- An object is created using curly brackets, and values are added as properties with a colon separating the property name and value.
- The console.log method is used to display the object and its properties in the console.
- Dot notation is used to access and change values within an object.
- Bracket notation is introduced as an alternative to dot notation, useful for properties with special characters or dynamic property names.
- Nested objects are explained, where an object can contain other objects as values.
- Functions can be stored in objects as methods, and examples like console.log and Math.random are given.
- Built-in objects like JSON and localStorage are introduced to handle data conversion and storage.
- The JSON object is used to convert JavaScript objects to JSON strings and vice versa.
- LocalStorage is used to save values more permanently, even after refreshing the page.
- A practical example is given by adding a score feature to a rock-paper-scissors game using objects.
- The lesson explains how to update and display the score, and how to reset it using localStorage.
- The concept of object references is introduced, explaining that objects are stored in memory and variables hold references to them.
- Shortcuts like destructuring, shorthand properties, and shorthand methods are introduced to simplify working with objects.
- The lesson concludes with exercises to practice working with objects.
9 Document Object Model (DOM)
- The lesson begins with an introduction to the Document Object Model (DOM) and its importance in JavaScript for manipulating web pages.
- A new HTML file is created, and existing code is copied into it to set up the environment for learning about the DOM.
- The document object is introduced as a built-in object in JavaScript that represents the web page, allowing manipulation of its elements.
- Examples are provided to demonstrate how changing properties of the document object, like 'innerHTML' and 'title', directly affects the web page.
- The syntax for accessing and modifying properties of the document object using dot notation is explained in detail.
- The lesson covers how HTML elements are converted into JavaScript objects when accessed through the DOM, allowing for manipulation using properties and methods.
- The 'document.querySelector' method is introduced for selecting any HTML element from the page and manipulating it within JavaScript.
- Practical examples are given, such as creating a button and changing its text using 'innerHTML' and 'document.querySelector'.
- The concept of class selectors in 'querySelector' is explained, showing how to select specific elements using class names.
- The lesson includes a project-based approach, demonstrating how to use the DOM to create interactive web elements like a YouTube subscribe button and a rock-paper-scissors game.
- The importance of separating JavaScript code into functions for cleaner and more maintainable code is emphasized.
- The lesson also covers handling keyboard events using 'onkeydown' and the 'event' object to create interactive features like an Amazon shipping calculator.
- Additional details about JavaScript, such as type coercion and converting strings to numbers, are discussed to ensure proper manipulation of DOM values.
- The window object is introduced as another built-in object representing the browser, explaining its relationship with the document object and other browser features.
- The lesson concludes with a summary of the key concepts learned, including DOM manipulation, event handling, and the integration of JavaScript with HTML.
10 HTML, CSS, and JavaScript Together
- The lesson begins with an introduction to CSS, explaining that it is a language used to change the appearance of websites. It is one of the three core languages used in web development, alongside HTML and JavaScript.
- The instructor demonstrates how to create a new project by copying an existing HTML file and renaming it. This new project will incorporate CSS to enhance the visual design of the website.
- The first project involves styling a YouTube subscribe button. The instructor reviews CSS basics, such as using the style element and CSS selectors to target specific elements. They explain how to add multiple classes to an element and use CSS properties to style the button.
- The instructor provides a step-by-step guide on how to style the subscribe button, including changing the background color, text color, padding, font weight, and border radius. They also explain how to use the cursor property to change the mouse pointer when hovering over the button.
- The lesson covers how to change the CSS of an element when a button is clicked. The instructor explains how to use JavaScript to add and remove classes from an element, allowing for dynamic style changes based on user interactions.
- The second project involves styling an Amazon shipping calculator. The instructor demonstrates how to add classes to input elements and buttons, and then style them using CSS. They cover properties such as font size, padding, border, and cursor.
- The instructor introduces a shortcut for adding padding to multiple sides of an element using a single CSS property. This helps to simplify the code and reduce repetition.
- The third project focuses on styling a rock-paper-scissors game. The instructor explains how to load images into the project and use CSS to style buttons and other elements. They cover properties such as background color, border, width, height, and margin.
- The lesson includes a detailed explanation of how to use file paths to organize images and other assets in a project. The instructor demonstrates how to create folders and update the source paths in the HTML and CSS files.
- The instructor shows how to use JavaScript to dynamically update the content and styles of the rock-paper-scissors game based on user interactions. They explain how to insert HTML elements and update their attributes using JavaScript.
- The lesson concludes with a section on organizing code into separate files for HTML, CSS, and JavaScript. The instructor explains how to use the script and link elements to load external JavaScript and CSS files, making the code more modular and easier to manage.
- The instructor provides a summary of the key concepts covered in the lesson, including CSS basics, dynamic styling with JavaScript, and code organization. They encourage students to practice these concepts through exercises and further study.
11 Arrays and Loops
- The lesson begins with creating a new HTML file named '11-arrays-and-loops.html' and copying the previous lesson's content into it. The title is changed to 'arrays and loops', and all CSS, HTML, and JavaScript are removed to prepare for the new lesson.
- An array in JavaScript is introduced as a type of value that represents a list of other values. An example array is created with the numbers 10, 20, and 30, and it is stored in a variable named 'myArray'. The array is then logged to the console.
- Accessing specific values in an array is demonstrated using square brackets and an index number. The first value is accessed with index 0, the second with index 1, and so on. Changing a value in an array is also shown by assigning a new value to a specific index.
- The syntax rules for creating an array are explained: start with an open square bracket, end with a closed square bracket, and separate each value with a comma. Arrays can contain any type of value, including numbers, strings, booleans, and objects.
- The 'typeof' operator is used to show that arrays are a type of object in JavaScript. The 'Array.isArray()' method is introduced to check if a value is specifically an array. The 'length' property and 'push' method of arrays are explained, with examples of adding and removing values using 'push' and 'splice'.
- A practical example of creating a to-do list project is introduced. The project involves creating an HTML file and a separate JavaScript file. The JavaScript file contains an array to store to-dos, and functions to add to-dos to the array and display them in the console.
- Loops in JavaScript are introduced, starting with the 'while' loop. The 'while' loop runs code repeatedly as long as a specified condition is true. The loop variable, loop condition, and increment step are explained in detail.
- The 'for' loop is introduced as a shorter and more organized way to create loops. The 'for' loop combines the loop variable, loop condition, and increment step in a single line. An example is provided to count from 1 to 5 using a 'for' loop.
- The concept of looping through an array is explained. A 'for' loop is used to go through each value in an array and perform an action with each value. An example is provided to display each value in the console.
- The accumulator pattern is introduced as a technique to accumulate results while looping through an array. An example is provided to calculate the total of all numbers in an array by adding each number to an accumulator variable.
- Another example of the accumulator pattern is provided to create a new array where each number is doubled. The 'push' method is used to add the doubled numbers to the new array.
- The second version of the to-do list project is created, where to-dos are displayed on the page. The 'renderTodoList' function is introduced to generate HTML for each to-do and display it on the page. The function is called every time a new to-do is added.
- The process of generating HTML using JavaScript is explained. The three-step process involves saving data, generating HTML from the data, and making the website interactive. This process is used for creating websites with JavaScript.
11 (Part 2) Arrays and Loops
- The tutorial begins with the goal of creating a final version of a to-do list application. This version allows users to add tasks with due dates and delete tasks from the list.
- The first feature implemented is the delete button. The HTML for the to-do list is generated dynamically, and a delete button is added inside each task's paragraph element. The button is made functional using the onclick attribute, which calls a JavaScript function to remove the task from the array and update the display.
- Next, the tutorial covers adding a due date feature. An input element of type 'date' is added to the HTML. The to-do list is updated to store tasks as objects with 'name' and 'due date' properties. The JavaScript code is modified to handle these objects, including updating the render function and the add task function.
- The tutorial introduces destructuring, a shortcut for extracting properties from objects. This technique is used to simplify the code that handles the to-do objects.
- The tutorial then moves on to styling the to-do list using CSS. A CSS grid is used to layout the tasks in a grid format. The inputs and buttons are styled to match the final design, including setting font sizes, colors, and spacing.
- The tutorial explains how to vertically align elements in the grid and how to ensure the add button stretches to match the input fields. It also covers adding margin and padding to elements for better spacing.
- The tutorial concludes with a review of arrays and loops in JavaScript. It explains that arrays are references, meaning multiple variables can point to the same array in memory. It also covers the slice method for copying arrays and the destructuring syntax for extracting values from arrays.
- The tutorial discusses the break and continue statements in loops. Break exits a loop early, while continue skips the current iteration and moves to the next one. Examples are provided using both for and while loops.
- Finally, the tutorial demonstrates how to use loops within functions. It shows how to create a function that processes an array and returns a new array, and how to use the return statement to exit a loop early within a function.
12 Advanced Functions
- The lesson begins with creating a new file named '12-Advanced Functions.html' and copying content from '11 Arrays and Loops' into it. The title is changed to 'Advanced Functions', and previous JavaScript code is removed.
- A basic function named 'greeting' is created, which logs 'hello' to the console. The concept of calling or running a function is introduced.
- Functions are explained as values, similar to numbers, strings, and booleans. A function can be saved in a variable, demonstrated by creating a variable 'functionOne' that holds a function logging 'hello 2'.
- Anonymous functions are introduced, which are functions without a name. The lesson explains that the traditional function syntax is a shortcut that offers readability and hoisting, allowing functions to be called before they are defined.
- Functions can be stored in objects, making them methods. An example is given where a function is saved in an object property and accessed using dot notation.
- The concept of passing functions into other functions as parameters is introduced, known as callback functions. An example is provided where a function is passed into another function and called within it.
- The 'setTimeout' function is introduced, which runs a function after a specified time. The concept of asynchronous code is explained, where the code does not wait for the timer to finish before moving to the next line.
- The 'setInterval' function is introduced, which repeatedly runs a function at specified intervals. The lesson explains how to stop the interval using 'clearInterval' and the interval ID.
- A practical example is provided where an 'autoplay' button is added to a rock-paper-scissors game, using 'setInterval' to make the computer play every second. The button toggles the autoplay feature on and off.
- The 'forEach' method is introduced as a preferred way to loop through arrays, providing better readability than traditional loops. An example is given where 'forEach' is used to log array values and their indices.
- A practical example is provided where 'forEach' is used to display a to-do list on a webpage, replacing a traditional for loop.
- The lesson explains how to skip iterations in 'forEach' using 'return' statements, similar to 'continue' in traditional loops. It also notes that 'forEach' does not support 'break' statements, recommending traditional loops if early exit is needed.
12 (Part 2) Advanced Functions
- Arrow functions provide a shorter syntax for writing functions in JavaScript. They use an arrow (=>) instead of the 'function' keyword and curly brackets.
- Arrow functions can be called in the same way as regular functions by using the variable name followed by parentheses.
- Parameters in arrow functions are defined within parentheses, similar to regular functions. If there is only one parameter, the parentheses are optional.
- Arrow functions can have implicit returns. If the function body contains only one expression, the curly brackets and 'return' keyword can be omitted.
- Arrow functions are particularly useful when passing functions as arguments to other functions, such as in array methods like 'forEach'.
- In some cases, regular functions are preferred over arrow functions, such as when function hoisting is needed or for better readability.
- The 'addEventListener' method is recommended over the 'onclick' attribute for adding event listeners to elements. It allows for multiple listeners and better control.
- The 'filter' array method creates a new array with elements that pass a test implemented by a provided function.
- The 'map' array method creates a new array populated with the results of calling a provided function on every element in the calling array.
- Closures in JavaScript allow a function to retain access to its lexical scope, even when the function is executed outside that scope.
13 Start the Amazon Project and Intro to Git
- The lesson begins with an introduction to the Amazon Project, an e-commerce website similar to Amazon, where users can add products to a cart, view the cart, and track orders.
- The first step is to download the starting code for the project from GitHub, which includes all necessary HTML and CSS files. This is done to focus on JavaScript rather than HTML and CSS.
- The downloaded code is then opened in a code editor (VS Code), and the project is set up to be viewed in a browser using Live Server.
- The lesson emphasizes the importance of JavaScript in making the website interactive, as HTML and CSS alone can only create the visual structure.
- The next part of the lesson introduces Git, a version control system that helps track changes in code. Instructions are provided for installing Git on both Windows and Mac.
- The Git repository is initialized in the project folder, and a username and email are configured for Git. Changes are then committed to Git to start tracking them.
- The lesson demonstrates how to use Git to track changes, undo changes, and view the history of changes in the code.
- The main idea of JavaScript is introduced: saving data, generating HTML, and making the website interactive. This is demonstrated by creating a list of products on the homepage using JavaScript.
- The data for the products is saved in a JavaScript array, and a loop is used to generate HTML for each product. The generated HTML is then inserted into the webpage using the DOM.
- The lesson explains how to make the 'Add to Cart' button interactive by using event listeners and data attributes to identify which product is being added to the cart.
- A cart array is created to store the products added to the cart, and the quantity of each product is managed to ensure that duplicate products are not added multiple times.
- The lesson also covers how to calculate the total quantity of products in the cart and display this quantity on the webpage.
- Finally, the lesson reviews the changes made to the code using Git, commits the changes, and emphasizes the importance of using Git for version control in larger projects.
14 Modules
- The text introduces JavaScript modules as a way to organize code and prevent naming conflicts. It starts by explaining the problem of using multiple script tags, which can lead to naming conflicts when the same variable name is used in different files.
- An example is given where a variable named 'cart' is declared in both 'cart.js' and 'amazon.js', causing an error. The solution is to use modules, which contain variables within a file, preventing conflicts with variables in other files.
- To create a module, the script tag for 'cart.js' is removed from 'amazon.html'. This prevents the 'cart' variable in 'cart.js' from being declared globally, avoiding conflicts.
- The text explains the steps to use modules: add a 'type="module"' attribute to the script tag, export variables from the module file, and import them into the file where they are needed.
- An example is provided where the 'cart' variable is exported from 'cart.js' and imported into 'amazon.js'. The import statement includes the file path to locate 'cart.js'.
- The text also covers the benefits of modules, such as avoiding naming conflicts and not having to worry about the order of script tags. Modules allow importing only the necessary variables, making code management easier in large projects.
- The text demonstrates how to use modules to organize code by moving functions related to the cart into 'cart.js' and importing them into 'amazon.js'. This practice groups related code together, improving code readability and maintainability.
- The text explains how to handle naming conflicts when importing variables by using the 'as' keyword to rename imported variables, ensuring no conflicts occur within the importing file.
- The text also covers the use of live server to ensure modules work correctly, as modules do not work when opening HTML files directly in the browser.
- Finally, the text provides a detailed example of creating a checkout page using modules, including generating HTML with JavaScript, making the page interactive, and saving the cart data in local storage to persist between page reloads.
15 External Libraries
- External libraries are pieces of code written by other developers and made available on the internet. They can be loaded into your project to save time and avoid duplicating work.
- To load an external library, you add a script tag with the source attribute pointing to the URL of the library. This allows the code from the URL to be used in your project.
- An example of loading an external library involves creating a function in the external code and then calling that function in your project to see its effect.
- External libraries are often minified to reduce their size, making them load faster. Minification removes extra spaces and shortens variable names.
- The DJ.js library is an example of an external library that helps with date manipulation. It provides methods to get the current date, add days to a date, and format dates in a readable way.
- Using external libraries can simplify complex tasks, such as date calculations, by leveraging pre-written code. This saves time and ensures reliability.
- JavaScript modules can be used to load external libraries in a way that avoids naming conflicts. The ESM (ECMAScript Module) version of a library is designed to work with JavaScript modules.
- To use an ESM version of a library, you use the import statement with the URL of the library. This method keeps the code contained within a module, preventing conflicts.
- Default exports in JavaScript modules allow you to export a single value or function from a file, making the import syntax cleaner.
- The MVC (Model-View-Controller) design pattern is a way to organize code by separating data management (Model), UI rendering (View), and user interaction handling (Controller).
- In the context of the checkout page example, the Model manages the cart and delivery options data, the View generates the HTML for the order and payment summaries, and the Controller updates the data and regenerates the HTML when user interactions occur.
- Using MVC ensures that the UI always reflects the current state of the data, making the code more maintainable and less error-prone.
16 Testing
- If you encounter an error in your project but your code seems correct, it might be due to bad data in local storage. Run 'localStorage.clear()' in the console to clear it and refresh the page.
- When saving data to local storage, always use 'JSON.stringify' to avoid errors like '[object Object]'.
- Manual testing involves opening the website and trying out the code to see if it works. For example, checking if prices displayed using the 'formatCurrency' function are correct.
- Manual testing is useful for quick checks but has disadvantages: it's hard to test every situation and retest the code after changes.
- Automated testing uses code to test code, saving time and effort. It involves writing test scripts that automatically check if the code works as expected.
- To create an automated test, group test code in a folder, create test files, and write code to test functions. For example, testing 'formatCurrency' with different values to ensure it works correctly.
- Automated tests can easily test multiple situations (test cases) and retest the code after changes, unlike manual testing.
- Test cases can be basic (checking if code works) or edge cases (testing tricky values). Both types are important for thorough testing.
- Naming test cases and grouping related tests (test suites) make it clear what is being tested and which tests pass or fail.
- Automated tests are saved and run using HTML files that load the test scripts, making it easy to rerun tests and check results in the console.
Testing Frameworks
- The text introduces the concept of a testing framework, which is an external library that helps write automated tests more easily.
- A testing framework, such as Jasmine, automates the creation of test suites, test cases, and the comparison of values, displaying results in the console.
- The tutorial demonstrates how to load Jasmine into a project by downloading and extracting the Jasmine standalone zip file, and then integrating it into the project structure.
- The process of creating a test suite and test cases using Jasmine is explained, including the use of functions like 'describe', 'it', and 'expect' to organize and execute tests.
- The tutorial shows how to run tests using Jasmine by opening a spec runner HTML file in a live server, which displays the test results in a web interface.
- The text explains how to write tests for specific functions, such as 'format currency', using Jasmine's syntax to compare expected and actual values.
- The tutorial covers the concept of mocking, which involves creating fake versions of methods to control their behavior during tests, ensuring consistent and reliable test results.
- The process of organizing test files to match the structure of the code being tested is discussed, including the creation of folders and proper naming conventions.
- The tutorial demonstrates how to test more complex functions, such as 'add to cart', by creating tests for different conditions and using mocks to control dependencies like local storage.
- The text explains the importance of test coverage, which measures how much of the code is being tested, and the goal of maximizing test coverage by testing all conditions of an if statement.
- The tutorial introduces integration tests, which test multiple units of code working together, and shows how to create such tests for functions that render parts of a web page.
- The text explains how to use hooks in Jasmine, such as 'beforeEach' and 'afterEach', to run setup and cleanup code before and after each test, reducing duplication and improving test organization.
- The tutorial concludes with a summary of the key concepts learned, including the use of Jasmine for automated testing, the creation of test suites and cases, mocking methods, and writing integration tests.
17 Object-Oriented Programming
- If you encounter an error in your project but your code looks correct, you may have bad data saved in local storage. To fix this, run 'localStorage.clear()' in your console to remove everything from local storage and then refresh the page.
- If you see an error like '[object Object]', it means you saved something directly into local storage without using 'JSON.stringify()'. Always stringify your data before saving it to local storage, then clear your local storage and refresh the page.
- Object-oriented programming (OOP) is another style of programming that organizes code into objects. Many programming languages use OOP, making it a useful skill to learn.
- In procedural programming, code is organized into separate functions. In OOP, code is organized into objects, grouping data and functions together.
- To convert procedural code to OOP, create an object and move related data and functions into it. For example, in a shopping cart application, you can create a 'cart' object and move all cart-related data and functions into this object.
- When moving functions into an object, convert them into methods by removing 'export' or 'function' keywords and using shorthand method syntax. Use 'this' to refer to the object within its methods.
- OOP represents real-world objects as digital objects. For example, a physical shopping cart can be represented as a JavaScript object with properties (products) and methods (add or remove products).
- OOP makes it easy to create multiple objects. For example, you can create separate carts for personal and business purchases by copying the cart object or using a function to generate multiple cart objects.
- To avoid code duplication, use a function to generate objects. This function can take parameters to customize each object, such as different keys for local storage.
- Test the OOP code by logging the objects to the console and ensuring they work as expected. For example, check that adding products to the cart updates the cart object correctly.
- OOP helps in managing and organizing code better, making it more intuitive and easier to understand. It also allows for creating multiple instances of objects efficiently.
Classes
- Object-oriented programming (OOP) introduces the concept of Classes, which are designed to generate objects more efficiently than functions.
- To create a Class in JavaScript, use the 'class' keyword followed by the class name in Pascal case (e.g., 'Cart').
- Properties and methods are defined within the class. Properties are initialized with 'undefined' and methods are defined without commas.
- The 'Constructor' method in a class allows for setup code to run automatically when an object is generated, making the code cleaner and more organized.
- Private properties and methods, denoted by a hash (#) symbol, can only be accessed within the class, enhancing code safety by preventing unintended modifications.
- The tutorial demonstrates converting a function-based object generation to a class-based approach, showing how to move properties and methods into the class.
- The 'new' keyword is used to generate objects from a class, ensuring each object has the defined properties and methods.
- The tutorial also covers converting existing objects into classes, using the 'map' method to loop through arrays and transform objects into class instances.
- Methods within classes can be used to encapsulate related code, making the overall codebase cleaner and more maintainable.
- The tutorial emphasizes the benefits of using classes, such as cleaner syntax, better organization, and additional features like constructors and private properties.
Inheritance
- Inheritance is a key feature of object-oriented programming that allows code reuse between classes.
- The tutorial begins by explaining the concept of inheritance using a project example with different types of products like clothing and appliances.
- Clothing and appliances are specific types of products that share common properties like name, price, and rating but also have unique properties such as a size chart for clothing.
- Instead of duplicating code for each specific product type, inheritance allows a new class (e.g., Clothing) to inherit properties and methods from a parent class (e.g., Product).
- The parent class is referred to as the 'Product' class, and the child class is referred to as the 'Clothing' class.
- The tutorial demonstrates how to implement inheritance in code using the 'extends' keyword to create a Clothing class that inherits from the Product class.
- A new Clothing object is created, and its properties and methods are inherited from the Product class, which is verified using console.log.
- The tutorial explains how to add specific properties to the Clothing class, such as a size chart link, using a constructor and the 'super' keyword to call the parent class's constructor.
- The tutorial also covers method overriding, where a method in the child class can replace a method in the parent class, and polymorphism, which allows methods to be used without knowing the exact class type.
- Examples are provided to show how inheritance and polymorphism can make code cleaner and more efficient.
- The tutorial concludes with a summary of the key points learned about inheritance, method overriding, and polymorphism, and encourages further practice through exercises.
18 Intro to Backend, Callbacks, Async Await
- The backend is a separate computer that manages the data of a website. For example, in the Amazon project, the backend computer owned by Amazon receives order information from the user's computer, which is the frontend.
- HTTP (Hypertext Transfer Protocol) is used for communication between the frontend and backend. An HTTP message can carry information such as an Amazon order. When the backend receives this message, it processes the order.
- To send an HTTP message using JavaScript, create a new file for practice. Use the built-in class `XMLHttpRequest` to generate an HTTP request object. Set up the request with the `open` method, specifying the type of request (e.g., GET) and the URL of the backend. Finally, send the request using the `send` method.
- A URL (Uniform Resource Locator) is like an internet address that helps locate another computer. It consists of the protocol (e.g., HTTPS) and the domain name (e.g., amazon.com). The browser can display different types of responses (e.g., text, JSON, HTML, images) based on the URL path.
- The `XMLHttpRequest` object can handle asynchronous code, meaning it doesn't wait for the request to complete before moving to the next line of code. Use the `addEventListener` method to wait for the response and then access it using the `response` property.
- The request-response cycle involves sending a request to the backend and receiving a response. The response can be accessed in the code and used as needed. Different URL paths can provide different responses, and unsupported paths will result in an error.
- The backend API (Application Programming Interface) lists all supported URL paths and their responses. The backend can respond with various data types, including text, JSON, HTML, and images. The browser can display these responses in a more useful way than raw data.
- To integrate backend communication in a project, create a function to load data from the backend and use callbacks to handle asynchronous responses. This ensures that the code waits for the response before proceeding.
- Testing with a backend involves using hooks like `beforeAll` to load data before running tests. The `done` function ensures that the tests wait for the backend response before continuing. This approach can be used in various testing hooks to handle asynchronous backend communication.
Promises and fetch
- Promises provide a better way to handle asynchronous code compared to callbacks. They allow us to wait for asynchronous code to finish before proceeding to the next step.
- To create a promise, we use the 'new Promise' syntax, which requires a function that runs immediately. This function receives a 'resolve' parameter, which is used to indicate when the asynchronous operation is complete.
- In the example, a promise is created to load products asynchronously. Once the products are loaded, the 'resolve' function is called to proceed to the next step.
- Promises help avoid the problem of nested callbacks, which can make code difficult to read and maintain. By using promises, we can keep our code flat and more manageable.
- The '.then' method is used to add a next step to a promise. This method takes a function that runs after the promise is resolved.
- The 'Promise.all' method allows us to run multiple promises simultaneously and wait for all of them to finish before proceeding to the next step. This can make our code more efficient.
- The 'fetch' function is introduced as a better way to make HTTP requests. Unlike 'XMLHttpRequest', 'fetch' returns a promise, making it easier to handle asynchronous operations.
- The tutorial demonstrates converting existing callback-based code to use promises and 'fetch', showing how promises can simplify the code and make it more readable.
- The 'resolve' function in a promise can also pass values to the next step, allowing for data sharing between steps.
- The tutorial concludes with examples of using promises and 'fetch' in a project and tests, highlighting the benefits of using promises for asynchronous code handling.
Async Await
- The tutorial begins by explaining the limitations of using promises for handling asynchronous code, such as the need for extra code like creating new promises and using resolve.
- Async Await is introduced as a shortcut for promises, which simplifies the code by removing the need for extra steps. The first example involves creating a function named loadPage and using async in front of the function to make it return a promise.
- The tutorial demonstrates how to call the loadPage function and use .then to handle the next step, showing that async makes the function return a promise and simplifies the code.
- The tutorial explains that returning a value from an async function converts it into a resolved value, which can be accessed in the next step. This is demonstrated by returning a string value and logging it to the console.
- The tutorial introduces the await keyword, which allows the code to wait for a promise to finish before moving to the next line. This is demonstrated by using await to wait for a function that fetches products from the backend.
- The tutorial shows how to use async await to load products, load the cart, and render the page in a cleaner and more readable way compared to using promises.
- The tutorial explains that await can only be used inside an async function and demonstrates how to handle errors using try-catch blocks with async await.
- The tutorial covers error handling for callbacks, promises, and async await, showing how to display error messages and handle unexpected errors.
- The tutorial demonstrates how to manually create errors using throw and reject, and explains the difference between synchronous and asynchronous error creation.
- The tutorial explains how to use async await in a project to load products, load the cart, and render the page, making the code cleaner and more readable.
- The tutorial covers how to handle errors in async await using try-catch blocks and demonstrates how to manually create errors in promises using throw and reject.
- The tutorial explains how to use URL parameters to save data in the URL and retrieve it using JavaScript, which is useful for creating dynamic pages like a tracking page.
- The tutorial demonstrates how to add URL parameters to links and retrieve them using the URL class and searchParams method in JavaScript.
- The tutorial concludes by summarizing the key points covered, including the use of async await, error handling, and URL parameters.
Next steps after this course
- The next step after this course is to learn how to create your own backend.
- To create a backend, you need to learn the command line.
- After mastering the command line, you should learn Node.js, a technology that allows backend creation.
- You can also learn how to put a website on the internet using a specific video provided.
- Exercises are available to help practice what was learned in the lesson.
- Support the channel by liking, subscribing, and sharing the video.
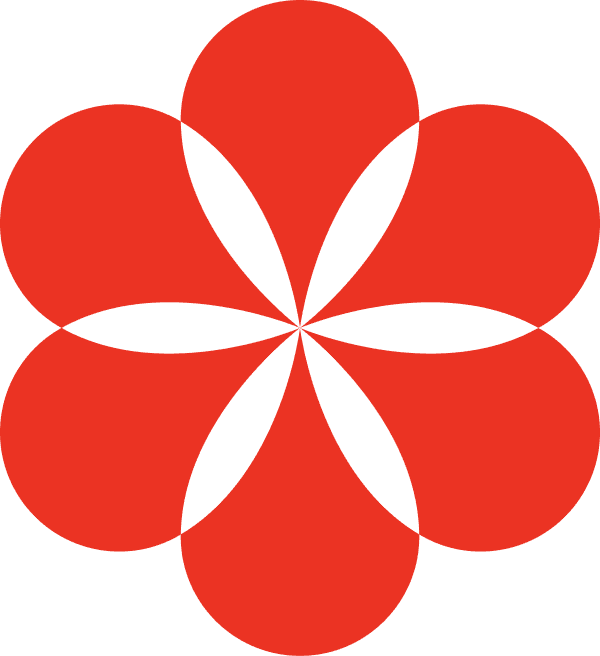
Turn any content into your knowledge base with AI
Supamind transforms any webpage or YouTube video into a knowledge base with AI-powered notes, summaries, mindmaps, and interactive chat for deeper insights.