Crafting a Role-Based File Storage System
InfoThis is a summary of the following YouTube video:
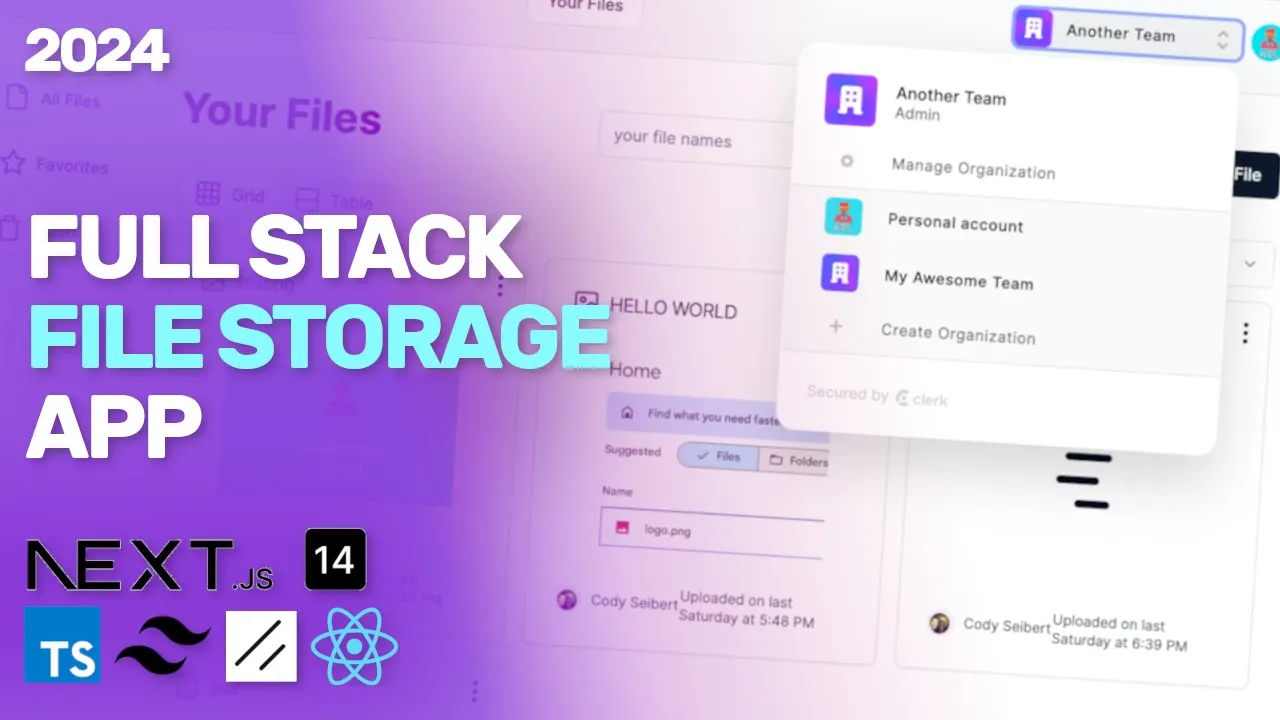
Build a File Storage App with Role Based Authorization (Next.js, Shadcn, Typescript)
Web Dev Cody
Feb 28, 2024
ยท
Education
Overview
- The tutorial is about building a full stack file storage application similar to Google Drive, featuring file upload, management, and role-based authorization.
- Authentication is handled using Clerk, and Convex is used for the backend as a service, both of which are sponsors of the video.
- The application includes a dashboard where users can upload files, with error handling for missing titles, and a UI built using ShadCN and Next.js.
- Users can search files by name, switch between grid and table views, and filter files by type, such as images, CSV, and PDF.
- A Convex cron job runs every minute to delete files marked for deletion, but users can restore files before they are permanently deleted.
- The application allows users to mark files as favorites, which can be viewed in a separate favorites section, and files can be downloaded directly from the app.
- The app supports organizational accounts, allowing users to switch between personal and team-based accounts, with role-based permissions for file management.
- Role-based authorization is implemented to restrict actions based on user roles, such as allowing only admins to delete files.
- Users can manage their accounts, including updating profile pictures, with changes reflected in real-time due to Convex's real-time database capabilities.
- The tutorial emphasizes the use of websockets in Convex for live updates, enhancing the user experience with real-time data changes.
Next + Convex Setup
- The tutorial begins with creating a new repository named 'file Drive' and making it public under the MIT license. This allows for commits throughout the tutorial, enabling users to revisit specific parts or skip setup steps if they are more advanced.
- The project setup involves cloning the 'file Drive' repository into a workspace folder and opening it with VSS code. The initial step is setting up a Next.js project using the command 'npx create-next-app@latest', with default settings for TypeScript and Tailwind.
- Convex, an npm package, is installed next. The project is initialized with 'npx convex dev', which may require signing in if it's the first time. The project is then associated with the 'web dev Cod' team, and it appears on the Convex dashboard for further development.
- A Convex client provider is created inside the source app directory. The code is copied and pasted to wrap all components, allowing access to Convex hooks. The provider is imported and used in the layout, ensuring the app can connect to the Convex backend.
- The environment variable 'NEXT_PUBLIC_CONVEX_URL' is verified to ensure the React app can connect to the Convex backend for queries and mutations. The Next.js app is then run using 'npm run dev', and the setup is confirmed by accessing the app on localhost:3000.
Clerk Setup
- The tutorial focuses on setting up authentication using Clerk in a full-stack file storage application built with Next.js and Convex.
- To begin, the Clerk setup guide for Next.js is followed, starting with creating a new project named 'file drive' on the Clerk dashboard, using Google and email for authentication.
- Various social media sign-in options are available in Clerk, which can be easily integrated if needed in the future.
- API keys from Clerk are copied into the local environment configuration file (EnV local) for integration with Convex.
- A first-class integration between Clerk and Convex is utilized by copying the issuer key from Clerk and applying it in Convex's configuration file (auth.conf.ts).
- Clerk is used for both front-end authentication (e.g., showing or hiding widgets) and verifying API requests to ensure the user has a valid Clerk token.
- The Clerk Next.js package is installed instead of Clerk React, as the application is built with Next.js.
- The Convex client provider is wrapped with the Clerk provider, and necessary imports are made to integrate Clerk with Convex.
- Middleware is created to authenticate endpoints, with a matcher ensuring all endpoints require authentication, except those specified as public routes.
- Public routes are configured to allow access without redirection to a login page, ensuring a smooth user experience on landing pages.
ShadCN
- ShadCN is a component library favored for its ease of use and fun integration into applications. It allows developers to install components using a CLI command, making it straightforward to incorporate into projects.
- To set up ShadCN, you can skip the initial setup command if you already have a Next.js project. Installation involves running a command that prompts a questionnaire, where you can choose default styles and CSS variables.
- ShadCN offers a variety of components that can be easily added to your application. The library provides examples of fully worked-out applications, allowing developers to view and integrate code directly from GitHub repositories.
- The process of adding a component, such as a button, involves running a command that imports the component into a designated directory. This simplifies the integration of UI elements into your project.
- Verification of ShadCN's setup involves adding a component to your main page, such as a button, and ensuring it displays correctly. This confirms that ShadCN is properly integrated and functional within your application.
Clerk Components
- Clerk provides pre-built components for seamless integration with Next.js, allowing developers to easily implement authentication features without extensive coding. These components include a sign-in button that automatically triggers a modal for user authentication.
- The sign-in process can be customized to include options like signing in with Google, and upon successful authentication, users are redirected back to the homepage. This process is streamlined by Clerk's built-in functionalities.
- To manage user sessions, developers can use the 'useSession' hook, which requires conversion to a client component. This hook helps in determining the user's authentication status and dynamically displaying content based on whether the user is signed in or out.
- Clerk offers 'SignedIn' and 'SignedOut' components to conditionally render UI elements based on the user's authentication state. These components simplify the logic needed to show or hide elements like sign-in or sign-out buttons.
- Styling of authentication buttons can be customized to match the application's design, such as using styles from ShadCN. This ensures that the authentication components blend seamlessly with the rest of the application's UI.
First Convex Mutation
- The tutorial focuses on building a full stack file storage application using Convex and Clerk, with features like file upload, management, and role-based authorization.
- Convex is used to handle data operations, including creating mutations and queries for interacting with the database.
- A mutation in Convex is an endpoint for modifying data, such as storing entries in the database, while queries are used for fetching data.
- The tutorial demonstrates creating a TypeScript file for handling file storage, where a mutation is defined to store file names in the database.
- The mutation allows passing arguments from the front end, with built-in validation in Convex for data types like strings.
- A button is added to the front end to trigger the mutation, demonstrating how to use Convex's useMutation hook for type-safe operations.
- The real-time capability of Convex is highlighted, showing automatic updates in the UI when data changes in the database.
Convex Query
- The tutorial introduces the concept of a query in the context of a full-stack file storage application. Queries are used to retrieve data from a database, as opposed to mutations which are used to create and store data.
- A query is defined using an export statement, taking an empty object as arguments and a handler context. The query fetches all entries from a specified table and returns them to the front end.
- To implement this on the front end, a function is called using a query API. This function retrieves data from the database and displays it in the UI. The example provided involves mapping over the retrieved files and displaying each file's name in a div element.
- The tutorial highlights the real-time data capabilities of Convex. When data is created or deleted, notifications are sent via WebSocket events to update all connected clients in real-time, demonstrating the live data feature.
- Convex allows for schema definition, which enhances type safety by specifying the structure and required fields of database tables. This ensures that the front end knows exactly what data to expect from the back end.
Auth in Convex
- Authentication is crucial for backend operations in Convex to ensure that only logged-in users can create or fetch files. This is achieved by checking the user's identity through the context object in Convex, specifically using 'context.auth.getUserIdentity'.
- If the identity is defined, it indicates that the user is logged in and has been validated against Clerk, a third-party authentication service. If not, the user is considered not logged in.
- To prevent unauthorized file creation, the system throws a 'ConvexError' if a user without a valid identity attempts to create or upload a file. This ensures that only authenticated users can perform these actions.
- Similarly, when fetching files, the system checks for user identity. If no identity is found, it returns an empty array to prevent the UI from crashing, ensuring a smooth user experience even for unauthenticated users.
- The tutorial demonstrates setting up authentication in a full-stack application using Convex, Next.js, ShadCN, and Clerk, highlighting the importance of secure backend operations.
Header
- The tutorial focuses on enhancing the UI of a file storage application by adding a header that includes an avatar for user login status. This header is implemented across all pages by creating a header component in the layout file, styled with a border and centered using CSS properties.
- A user button from Clerk is integrated into the header, allowing users to view a dropdown menu for signing out and managing their account. This button also provides a modal for security configurations, such as updating or removing the user's image.
- The header is styled with flexbox properties to ensure elements are aligned properly, including a padding and background color to distinguish it from the body. The 'Hello World' placeholder is suggested to be replaced with a logo, named 'File Drive'.
- The tutorial introduces the concept of adding organizations to the application using Clerk's built-in organization support. An organization switcher component is added next to the user button, but requires enabling in Clerk's settings.
- Once organizations are enabled, users can create and manage organizations, inviting others via email. The tutorial demonstrates creating an organization named 'My Awesome Team', allowing multiple users to access shared files.
- Each organization is assigned an org ID, which is used to associate data within the application, enabling members to edit, modify, or delete files. Custom roles and permissions can be set up in Clerk to manage access levels within organizations.
- The tutorial concludes with a demonstration of viewing organization details, such as members and roles, through Clerk's interface, highlighting the admin's ability to manage organization settings.
Scope Files using OrgId
- The tutorial focuses on building a full-stack file storage application with features like file upload, management, role-based authorization, and more, using organizations to manage data complexity.
- To manage files by organization, a 'use organization' hook is used in the frontend to retrieve organization data, which is then passed to Convex to fetch files associated with a specific organization.
- The organization ID (org ID) is crucial for isolating data between different organizations. It is stored in the schema and required for file creation and retrieval processes.
- An index is created on the files table by org ID to enable efficient querying of files associated with specific organizations, enhancing performance.
- Handling cases where org ID might be null or undefined is important, especially for personal accounts. Logic is added to use user ID as a fallback when org ID is not available.
- The tutorial includes steps to refactor code to accommodate both organization and personal account scenarios, ensuring data is correctly associated and isolated.
- The process involves checking if organization and user data are loaded before proceeding with queries, ensuring dependencies are resolved before execution.
- The tutorial suggests using optional fields during development to avoid schema collisions and recommends clearing tables during rapid prototyping to simplify data management.
- The implementation allows switching between different organizations and personal accounts, ensuring data remains isolated and correctly associated with the respective org ID or user ID.
Clerk Webhooks
- The backend must be properly authenticated to ensure that users have access to the correct organization IDs. Simply checking if a user is logged in is insufficient for verifying access to specific organizations.
- One approach to verify user access is to make requests to the Clerk API for each request, but this can be inefficient and may lead to rate limiting as the application scales.
- An alternative approach is to use JWT tokens to track the active organization ID, but this method has limitations due to the OpenID spec requirements, which may require using unconventional fields.
- The recommended approach is to use webhooks from Clerk to update the schema when organizations or users are created, allowing for efficient access checks without constant API calls.
- Convex allows the creation of an HTTP endpoint to handle webhook requests, which must be verified using cryptographic signatures to ensure they are from Clerk.
- Convex supports different runtimes, and the svix package is used to sign and verify webhooks, requiring a Node.js runtime for certain actions.
- Internal actions in Convex are used to handle webhook data, ensuring that only authorized queries and mutations can access them.
- When a user is created in Clerk, a webhook event is sent to update the user table in the database with the token identifier and Clerk ID.
- The process involves creating a users table and defining mutations to handle user creation and organization membership updates.
- Webhooks are configured in Clerk to listen for specific events like user creation and organization membership creation, which trigger updates in the database.
- Environment variables are used to store webhook secrets for secure verification of incoming webhook requests.
- The system is tested by simulating user creation and organization membership events, ensuring that the database updates correctly with the new information.
- The overall goal is to authenticate users and verify their authorization to perform actions like file uploads to specific organization IDs.
OrgId Authorization
- The tutorial focuses on creating a file storage application with features like file upload, management, and role-based authorization. It includes UI components such as dialogs, modals, and toasts.
- The process begins with setting up the environment using Next.js and Convex, followed by integrating Clerk for authentication and authorization purposes.
- A key part of the tutorial is verifying the OrgId when creating a file. This involves checking if the OrgId is associated with the user, using helper functions to streamline the process.
- The tutorial explains how to handle errors when a user does not have access to a specific OrgId, ensuring that unauthorized actions are blocked.
- It demonstrates the use of context and token identifiers to manage user identities and permissions within the application.
- The tutorial includes testing scenarios to ensure that unauthorized users cannot access or manipulate files associated with different OrgIds.
- A function is created to abstract the authorization logic, making it reusable for both queries and mutations within the application.
- The tutorial concludes with committing the changes made to implement OrgId authorization, ensuring that the application is secure and functions as intended.
File Upload Dialog
- The tutorial begins with setting up a full stack file storage application, focusing on creating a user-friendly interface with a title and upload button. The title is styled using CSS classes for text size and boldness, and the layout is adjusted using flexbox for alignment.
- A modal dialog is introduced for file uploads, utilizing the ShadCN library. The dialog allows users to input a file title and select a file. The tutorial explains how to install and use the dialog component, emphasizing the importance of importing from the correct directory.
- Form handling is implemented using React Hook Form and Zod for validation. The tutorial details setting up a form schema with title and file inputs, ensuring type safety and validation. It explains the use of useForm hook and Zod resolver for managing form state and validation.
- The tutorial covers handling file input changes and ensuring the file is correctly captured for upload. It discusses using a custom type for file validation and managing file input changes with an onChange event handler.
- The process of uploading files to Convex storage is explained. The tutorial describes creating a backend mutation to generate an upload URL, ensuring user authentication before allowing file uploads. It details the steps to call this mutation from the frontend and handle the file upload process.
- The tutorial includes steps to reset the form and close the modal dialog after a successful file upload. It explains using state management to control the dialog's visibility and resetting form fields to ensure a clean state for subsequent uploads.
- Throughout the tutorial, the importance of reading documentation and understanding the integration of various components and libraries is emphasized. The tutorial provides a comprehensive guide to building a file upload feature with a focus on user experience and backend integration.
Toast Component
- The tutorial focuses on implementing a toast notification component to provide user feedback when actions occur, such as file uploads.
- To add a toast, import the component into the layout file and set it up using the 'useToast' method, allowing it to display messages like 'file uploaded'.
- Customization of the toast component involves defining new color variants in the global CSS and Tailwind config, enabling changes like making the toast green for success messages.
- The tutorial demonstrates handling errors by showing a 'destructive' toast variant when a file upload fails, enhancing user feedback for unsuccessful actions.
- To improve user experience, the tutorial suggests resetting form values when modals are closed, ensuring old values do not persist when reopened.
Button Loader
- The tutorial focuses on enhancing user experience by implementing a button loader in a modal form. This ensures users receive feedback during submission delays.
- To achieve this, the form's state object is utilized to manage the button's disabled state, preventing multiple submissions during loading.
- The button is disabled using the form's state, specifically checking if the form is in a loading state to prevent further clicks.
- A spinner or loader is added to the button to visually indicate the loading process, using Lucid icons for a spinning effect.
- Adjustments are made to the button's styling, such as adding a flex gap to separate the spinner from the text, ensuring a polished look.
- The tutorial emphasizes the importance of polishing applications for better usability, even if it requires spending more time on details.
- The process concludes with committing the changes to the repository, highlighting the addition of the upload file dialog.
Styling Homepage
- The tutorial focuses on improving the homepage's appearance by offering two display options for files: a table or a collection of cards. A toggle feature is suggested to allow users to choose their preferred view.
- Before styling, the code undergoes refactoring to enhance structure and manageability. A new file, 'upload button TSX,' is created to encapsulate the upload button component, simplifying the main page code.
- Unnecessary code is removed to streamline the file, focusing on keeping only essential elements like the dialog and form submission logic. This results in a cleaner and more efficient codebase.
- The tutorial introduces a 'card component' to replace the existing list display of files. This involves importing necessary elements and creating a 'file card TSX' component to handle file display.
- The 'file card' component is designed to accept file properties, such as title and type, and includes a footer with a download button. The component is flexible to accommodate different file types.
- The layout is adjusted to display files in a grid format, enhancing visual appeal. Grid properties like column count and gap are configured to ensure a neat and organized presentation.
Delete File Dropdown
- The tutorial focuses on adding a delete file feature to a file storage application, emphasizing the importance of this functionality.
- A dropdown menu is used to facilitate file deletion, with the component imported and integrated into the file card actions.
- The delete action is highlighted with a trash icon, and the UI is adjusted for better alignment and spacing.
- A confirmation dialog is implemented to ensure users are certain about deleting a file, using an alert dialog component from ShadCN.
- The delete operation is linked to a Convex endpoint, which checks user authorization and file existence before deletion.
- Error handling is incorporated to manage cases where the file does not exist or the user lacks permission to delete it.
- A toast notification is added to inform users of successful file deletion, with adjustments made to the notification's appearance.
- The code is committed with a message indicating the addition of the delete file feature, marking progress in the tutorial.
Empty States
- The tutorial focuses on enhancing a file storage application's user interface by implementing an 'empty state' when no files are present. This involves displaying a placeholder image and message to prompt users to upload files.
- To achieve this, the tutorial suggests using open-source illustrations from a site like undraw, which offers Creative Commons images. The chosen image should match the application's theme, such as black and white for ShadCN.
- The process involves downloading an SVG image, adding it to the project's public folder, and using a Next.js image component to display it. The image is set with specific dimensions and an alt text for accessibility.
- The logic to display the image only when no files are present is implemented using a conditional check. If the files array is undefined or empty, the image and a prompt message are displayed.
- The tutorial emphasizes using CSS classes to style the UI, such as centering the image and text using flexbox properties. This ensures the UI remains user-friendly and visually appealing.
- Additional UI enhancements include adding an upload button below the message, which is conditionally displayed based on the presence of files. This button allows users to easily upload new files.
- The tutorial demonstrates how to dynamically update the UI when files are uploaded or deleted, ensuring the 'empty state' and file list are correctly displayed based on the current state of the file system.
Page Spinner
- The initial page load currently lacks a visual indicator, resulting in a blank screen, which can be improved for better user experience.
- To address this, a spinner can be displayed when files are undefined, indicating that content is loading.
- The spinner implementation can be borrowed from the existing button loader, and its size can be adjusted to 24 or 32 for better visibility.
- A 'Loading' text can be added below the spinner, centered on the page, with a gray text color to make it less intense than black.
- The spinner should only be shown when files are undefined, and the page can be refreshed to test this functionality.
- For a more seamless experience, server-side rendering can be considered to prefetch data, reducing the need for a full-page spinner.
- The code can be cleaned up by defining a constant 'isLoading' to check if files are undefined, improving readability and maintainability.
File Types
- The tutorial focuses on building a full stack file storage application with features like file upload, management, and role-based authorization.
- The application supports different file types such as images, CSVs, and PDFs, and the tutorial emphasizes the importance of tracking these file types for better display and management.
- A schema is created to define file types using a union in Convex, allowing for shared type validation between the schema and mutations.
- The front end is set up to pass the file type during uploads, using a map to convert MIME types to user-friendly labels like 'image', 'PDF', and 'CSV'.
- The tutorial demonstrates how to handle errors related to file type validation and how to ensure the Convex runner processes files correctly.
- Icons are used to represent different file types in the UI, with a lookup map to associate file types with specific icons.
- The tutorial includes steps to display image previews for image files and discusses potential enhancements for displaying previews of other file types.
- A utility function is created to generate URLs for accessing stored files, and Next.js configuration is updated to allow image loading from specific domains.
- The UI is adjusted to improve the display of file icons and previews, ensuring a consistent and visually appealing layout.
Download Button
- The tutorial explains how to implement a download button that opens a new tab to access file locations on Con X. This feature allows users to download files directly or preview them in a new tab.
- The process involves handling different file types such as images, PDFs, and CSVs. Images are displayed in a new tab, PDFs open in a previewer, and CSVs are downloaded directly.
- The tutorial notes that this functionality may not work consistently across all browsers, suggesting a need for testing and verification on different platforms.
- The final step in the tutorial involves committing the changes to the codebase, with a commit message indicating the addition of image preview capabilities and file download functionality.
Search Bar
- The tutorial focuses on adding a search bar to a file storage application, allowing users to filter files by title.
- A new component, 'search bar', is created using TypeScript, which includes a form with an input box and a search button.
- The form schema is set up to track search criteria, with the default query set to an empty string.
- The search bar is integrated into the UI, initially placed under the page header, and later moved to the header for better layout.
- Flexbox is used to align the search bar components horizontally, and unnecessary labels are removed for a cleaner interface.
- The search functionality is implemented by modifying the Convex query to filter files based on the search input.
- State management is handled using React's useState hook to keep track of the search query.
- The backend logic is adjusted to perform case-insensitive searches by converting both file names and queries to lowercase.
- UI improvements include resizing the search button for better aesthetics and ensuring the search bar is always visible.
- The tutorial addresses handling empty states and refactoring code for better user experience and cleaner logic.
Side Nav
- The text discusses the addition of a side navigation feature to a web application, emphasizing its importance for functionalities like favoriting or starring documents. This feature is initially added directly to the page, but the possibility of using Next.js layouts for nested navigation is mentioned.
- The author prefers a simple initial implementation, planning to refactor later. A basic side navigation is created using a div element, with a 'testing' label to ensure visibility. The layout is split into left and right sections using a flex wrapper, with the side navigation on the left and main content on the right.
- The side navigation is given a fixed width, initially set to 32 units and adjusted to 40 for better appearance. The layout is expanded to fill the full page width, acknowledging some loss of horizontal space due to the side navigation.
- A button is added to the side navigation for viewing all files, with plans to convert buttons to links later. The button includes a file icon and is styled with flex and gap properties to ensure proper spacing and alignment.
- The text describes the process of aligning the button to the left and adjusting spacing using flex properties. A gap of eight units is set between elements for better visual separation.
- A link for 'favorites' is added to the side navigation, featuring a star icon. The text notes the need for additional spacing between buttons, achieved by setting a flex column layout with a gap of four units.
- The 'favorites' link is intended to lead to a new page, which is not yet created. The text concludes with a note on restructuring the code to support nested navigation.
Refactor Layout
- The tutorial involves creating a full stack file storage application with features like file upload, management, and role-based authorization.
- The focus is on setting up routes in Next.js, specifically for a dashboard that includes 'favorites' and 'all files' pages.
- The dashboard page will incorporate a layout where pages are passed as children, allowing for a structured navigation system.
- The process involves copying existing code, organizing it into a dashboard layout, and ensuring proper import of necessary components like buttons and icons.
- The files page is simplified by removing unnecessary elements, relying on the layout to handle common components.
- A blank 'favorites' page is created, with plans to add content similar to the files page, such as a title.
- Adjustments are made to import paths to ensure components are correctly linked after moving files.
- The navigation between the 'files' and 'favorites' pages is tested to ensure functionality, with plans to add more content to the 'favorites' page.
Active Link Style
- The tutorial focuses on creating a dynamic side navigation component in a Next.js application. This involves abstracting the navigation into a client component, which can be named 'side nav'.
- The component is created in a new file, sv.TSX, and is exported as a function. The 'use client' directive is used to ensure the component is client-side rendered.
- The component uses the 'usePathname' hook from Next.js to determine the current path. This allows the application to style navigation links differently based on the active route.
- The 'clsx' library is introduced to manage conditional styling. It allows the application to apply different styles, such as changing text color, based on whether a navigation link is active.
- The tutorial demonstrates how to implement this by checking if the current path includes specific routes like 'dashboard files' or 'dashboard favorites'. If a route is active, the link is styled with a different color.
- The process involves testing and adjusting the component to ensure that the side navigation updates correctly as different links are clicked.
File Browser
- The discussion focuses on creating a shared component for the favorites page that can be reused across different pages. This involves abstracting common functionalities into a single component to handle different data queries.
- The idea is to create a common component that can be used on the dashboard, potentially replacing the current browser component. This involves moving most of the existing code into a new directory for better organization.
- The plan includes renaming the component to 'file browser' and using it across multiple pages. This allows for consistent functionality and appearance between the files and favorites pages.
- To implement this, the component will be modified to accept a title as a prop, allowing different pages to display different titles such as 'Your Files' or 'Favorites'.
- The main distinction between the pages will be the type of data query each uses, which will be passed into the shared component.
Mark as Favorite
- The tutorial focuses on adding a 'Mark as Favorite' feature to a file storage application, enhancing user interaction by allowing files to be marked as favorites through a dropdown menu.
- Initially, the dropdown menu is modified to include a 'Favorite' option alongside existing options like 'Delete'. The visual appearance is adjusted by removing the red color and adding a separator for better UI clarity.
- The core functionality involves creating a new table in the database schema to store favorite records, linking files to users and organizations. This setup allows for efficient management of favorite files across different user accounts and organizations.
- An index is added to the favorites table to facilitate quick lookups, using user ID, file ID, and organization ID as keys. This indexing strategy optimizes the process of checking if a file is already marked as a favorite.
- A toggle function is implemented using Convex, which handles marking a file as a favorite or removing it from favorites. This function checks user access and file existence before performing database operations.
- The code is refactored to improve readability and reusability by creating utility functions. These functions handle access checks and database interactions, reducing code duplication and potential errors.
- Testing involves invoking the toggle function from the file card component, ensuring that clicking the 'Favorite' option correctly updates the database by adding or removing entries in the favorites table.
- The final steps include updating the UI to reflect the favorite status of files, such as filling the star icon for favorited files and displaying them in a dedicated favorites list.
Favorites Page
- The tutorial focuses on building a query system to retrieve files marked as favorites in a file storage application. It involves converting the file browser to an Ed client and running a query when the page loads.
- A new query function is proposed, which can either be a separate function or an argument added to an existing function to filter for favorite files. The argument is a Boolean indicating whether to retrieve only favorite files.
- The process involves querying the database to get all favorite files associated with a user and organization ID. If the user is not defined, the function returns the files without filtering.
- The files are filtered to include only those marked as favorites by checking if their file IDs are in the favorites list. This method is suitable for smaller datasets, with a note on potential adjustments for larger datasets.
- The implementation is tested by passing necessary parameters like org ID and favorites status, ensuring the system only displays favorite files. The code is structured to allow reuse across different pages by passing different options.
- The tutorial concludes with a successful test showing the system correctly displays and updates favorite files, demonstrating the flexibility and efficiency of the implemented solution.
Favorite Star
- The tutorial focuses on enhancing a file storage application by implementing a feature to visually indicate if a file is marked as a favorite. This involves changing the star icon to a filled-in version when a file is favored, making it easier for users to identify their favorite files.
- To determine if a file is favored, the application can retrieve a list of all favorite files for a user. This list is then used to check each file's status on the front end, allowing the star icon to be toggled accordingly.
- A new method, 'get all favorites,' is introduced to fetch all favorite files associated with a user and organization ID. This method checks for user access and returns an array of favorites, which is then used to update the UI.
- The code is refactored to reduce redundancy by creating utility functions that handle user access checks and favorite retrieval. This refactoring helps streamline the codebase and improve maintainability.
- The application uses a query to fetch favorite files and passes this data to the file cards, which display the favorite status. A computed property, 'is favored,' is used to determine if a file should display a filled or half-filled star icon.
- The UI is updated to include text labels 'Favorite' and 'Unfavorite' next to the star icon, providing clear feedback to users about the action they are performing when clicking the icon.
- The tutorial concludes with testing the implementation to ensure that the favorite status is correctly displayed and updated in the UI, confirming the functionality of the new feature.
Role Based Authorization
- The tutorial focuses on implementing role-based authorization in a file storage application, ensuring only admins or moderators can access certain features like the delete button.
- The process involves using Clerk to manage user roles within an organization, allowing only admins to perform specific actions such as deleting files.
- A Protect component from Clerk is used to hide UI elements based on user roles, ensuring that only users with the appropriate permissions can see and interact with certain features.
- The backend must also verify user roles to prevent unauthorized actions, even if a user attempts to bypass the UI restrictions by making direct network requests.
- Webhooks are utilized to track role assignments and updates, ensuring that the backend is aware of any changes in user roles within an organization.
- The tutorial demonstrates how to store and manage user roles in a database, using a schema that associates roles with organization IDs for fine-grained access control.
- Edge cases, such as handling personal accounts and ensuring at least one admin remains in an organization, are considered to maintain system integrity.
- The implementation includes updating user roles dynamically and ensuring that changes are reflected in both the frontend and backend, using webhooks to listen for role updates.
- Testing is conducted to ensure that role-based restrictions are enforced, with errors thrown when unauthorized actions are attempted, and successful actions when permissions are correct.
Delete Cron
- In file storage systems, files are not immediately deleted but marked for deletion, allowing a cron job to permanently delete them after a set period, such as 30 days.
- The tutorial suggests adding a trash subnavigation to view files marked for deletion, utilizing Convex's cron job capabilities to automate the deletion process.
- A new link called 'Trash' is added to the side navigation, leading to a trash dashboard where files marked for deletion can be managed.
- In the file browser, a filter is implemented to show only files marked for deletion, using a Boolean flag 'should delete' to identify these files.
- The schema is updated to include an optional Boolean 'should delete' to mark files for deletion, which the cron job will use to permanently delete files.
- When a file is marked for deletion, it is updated in the database with 'should delete' set to true, rather than being immediately removed.
- The user interface is adjusted to reflect the status of files marked for deletion, including changes to modals and navigation icons.
- Files marked for deletion are filtered out from the main file view, ensuring they only appear in the trash section until permanently deleted.
Restore Item
- The tutorial focuses on building a full stack file storage application with features like file upload, management, and role-based authorization.
- A method for restoring deleted files is introduced, allowing users to recover files marked for deletion by setting 'should delete' to false.
- The UI is updated to include a restore icon, possibly using an undo icon, and changes the text color to green for restored items.
- A cron job feature in Convex is utilized to automate file deletions, running actions or mutations at specified intervals, such as every minute.
- The cron job is designed to delete files marked for deletion by querying the database and removing both the storage files and their entries.
- The tutorial suggests handling large numbers of files by processing them in smaller batches to avoid timeouts during deletion.
- The cron job can be configured to run at different intervals, such as daily, and can notify users about scheduled deletions.
Upload User
- The tutorial focuses on enhancing a file storage application by adding user identification features, allowing users to see who uploaded files and when. This involves modifying the webhook to capture additional user information such as name and profile image during user registration.
- To implement this, the tutorial suggests updating the internal mutation to accept and store the user's name and image. This requires changes to the schema to include these fields, which are initially set as optional.
- The tutorial also covers handling user updates by creating a new internal mutation called 'update user'. This mutation fetches the user by their token identifier and updates their name and image in the database.
- To ensure the application responds to user updates, the tutorial instructs on subscribing to the 'user updated' event in Clerk, ensuring that any changes to user profiles are captured and reflected in the application.
- The tutorial addresses the issue of hardcoded values by suggesting the use of environment variables to store repeated values, improving maintainability and reducing redundancy.
- For displaying user information on file cards, the tutorial proposes fetching user profiles from the UI using a new query endpoint. This involves adding user IDs to files in the schema and ensuring the UI can access this data efficiently.
- The tutorial includes steps to integrate an avatar component for displaying user images and names on file cards, along with styling adjustments to improve the user interface.
- Finally, the tutorial demonstrates how to format and display the upload date using a date library, ensuring the information is presented clearly and accurately.
Minor Tweaks
- The tutorial focuses on refining the user interface and user experience of a file storage application. It begins by addressing the issue of overly long file names that extend beyond their designated space. The solution involves setting a base text size and normal font weight, with an option to truncate names exceeding 20 characters, adding ellipses for clarity.
- Icon sizes are standardized to ensure consistency across the application. The file icon is adjusted to match the size of other icons, using a class name to set the width and height to four units each.
- The tutorial emphasizes the importance of user experience by rearranging the order of actions. Downloading files is prioritized over marking them as favorites, reflecting its significance in the application's functionality.
- A logo is added to the application to enhance its branding. The logo is placed in the header, using the Next.js image component for optimal loading. The size is adjusted to ensure it is visible yet not overwhelming.
- Navigation improvements are made by ensuring the logo is clickable, redirecting users to the homepage. Additionally, a new link labeled 'Your Files' is added to the header, directing users to the dashboard or file management page.
- The tutorial suggests using a button with an outline variant for the 'Your Files' link, making it less intrusive while still clearly indicating its functionality.
- The session concludes with a commit to version control, summarizing the changes made, including logo and header updates, display of creation time and user avatars in cards, and repositioning of the download button.
Table
- The tutorial discusses the implementation of a data table for file management, highlighting the benefits of using a table view over a card view for certain applications. This approach can enhance user experience by allowing users to filter, sort, and perform actions like downloading or deleting files directly from the table.
- The tutorial introduces the use of a third-party library, TanStack React Table, to facilitate the creation of a data table with sorting features. The guide follows a step-by-step process to set up the table, including defining data and columns, and integrating it into the file browser.
- A toggle feature is suggested to switch between a file table and a file card view, enhancing the flexibility of the user interface. The tutorial explains how to define columns and pass data to the table, addressing potential TypeScript errors and refactoring needs.
- The tutorial details the customization of table columns, such as displaying the creation date and user information. It explains how to use custom functions to render specific data, like formatted dates and user profiles, within the table cells.
- An actions column is added to the table, allowing users to perform actions like downloading or deleting files. The tutorial describes how to extract and reuse components, such as file actions, to maintain code cleanliness and functionality across different parts of the application.
- The tutorial addresses the integration of favorite functionality within the table, ensuring that favorite files are correctly displayed and managed. It explains how to modify data structures to include favorite status and update the UI accordingly.
View Toggle
- The tutorial focuses on adding a view toggle feature to a file storage application, allowing users to switch between grid and table views. This enhances user experience by providing flexibility in how files are displayed.
- Tabs are introduced to facilitate the view toggle. The tutorial explains how to install and import necessary components, and how to integrate these tabs under the main header (H1) of the application.
- The grid and table views are placed within the tab contents, allowing users to switch between these views. The tutorial addresses issues with hardcoded widths and suggests removing them for better layout flexibility.
- Icons are added to the tabs for visual clarity, with adjustments made to styling using Flexbox to ensure proper alignment and spacing.
- The tutorial discusses persisting the user's view choice using local or session storage, although this is not implemented in the example.
- A select component is introduced to filter files by type (e.g., images, PDFs, CSVs). The tutorial covers installing the component, setting default values, and updating backend queries based on the selected file type.
- The tutorial emphasizes improving user experience by adjusting loading indicators to be more context-specific, such as changing 'loading your images' to 'loading your files' and positioning spinners appropriately.
- Accessibility improvements are suggested, such as adding labels to the select component and ensuring proper HTML attributes are used for better user interaction.
Personal Account Bug
- The issue addressed is the absence of a delete button for files in personal accounts, which is due to the current logic checking only for admin roles.
- The solution involves changing the logic to a condition that checks if the user ID of the file matches the current user's ID, allowing non-admin users to delete their own files.
- A new endpoint is created to fetch the current user's information using their Convex ID, not just the token identifier, ensuring accurate user identification.
- The process involves exporting a query function to retrieve user data based on the token identifier, returning null if no user is found, and providing the user object if successful.
- The front-end can now invoke this query to obtain the authenticated user's information, specifically the user ID, to compare with the file's user ID.
- The back-end logic is updated to replace the admin check with a 'can delete' condition, allowing file deletion if the user owns the file or is an admin.
- A utility function 'can delete file' is created to encapsulate the deletion logic, taking a user and a file as parameters, and determining access rights.
- This function is used in multiple places to ensure consistent logic application, reducing errors and improving maintainability.
- The changes are tested by uploading, deleting, and restoring files to ensure the new logic works correctly for both personal and admin accounts.
- The final step involves committing the changes with a message indicating the fix for the file deletion issue on personal accounts.
Footer & Landing Page
- The tutorial focuses on adding a footer to enhance the application's appearance. The footer is created using a new component, 'footer.TSX', with a specified height and background color. It includes links like 'Privacy Policy', 'Terms of Service', and 'About', styled with flexbox for alignment and hover effects.
- The landing page is improved by incorporating a pre-designed Tailwind component. The existing header is removed to avoid redundancy, and the landing page is customized with text promoting the application's features, such as easy file management.
- An icon representing the application is added to the landing page for branding purposes. Adjustments are made to ensure elements are centered and properly aligned, addressing issues with padding and layout.
- A 'Get Started' button is implemented to direct users to the dashboard. The button is linked using Next.js routing, ensuring users are redirected appropriately based on their authentication status.
- A bug is identified where the header is obscured by other elements. This is resolved by adjusting the header's z-index, ensuring it remains clickable and functional.
- The tutorial concludes with a commit of the changes and a note of thanks to the sponsors, Convex and Clerk, for supporting the tutorial. Viewers are encouraged to join the Discord channel for community interaction and support.
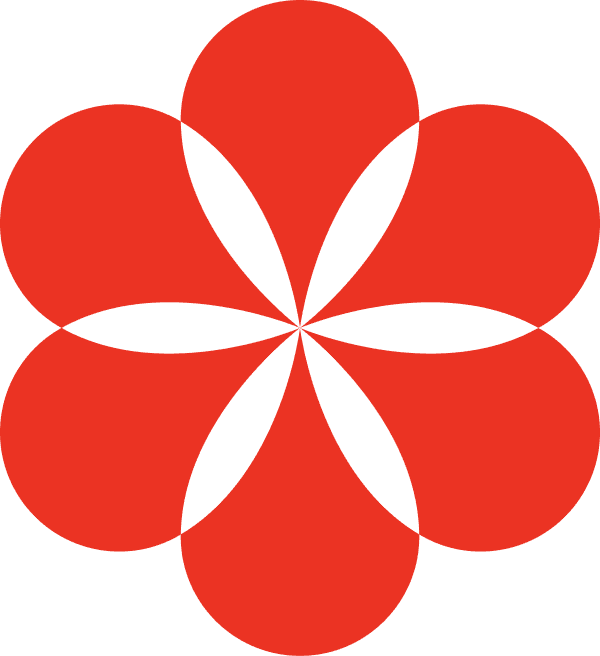
Turn any content into your knowledge base with AI
Supamind transforms any webpage or YouTube video into a knowledge base with AI-powered notes, summaries, mindmaps, and interactive chat for deeper insights.